Programming The ESP32 In C
Using The Espressif IDF
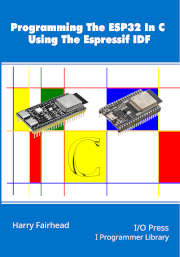
We have decided not to make the programs available as a download because this is not the point of the book - the programs are not finished production code but something you should type in and study.
The best solution is to provide the source code of the programs in a form that can be copied and pasted into a VS Code project.
The only downside is that you have to create a project to paste the code into.
To do this follow the instruction in the book.
All of the programs below were copy and pasted from working programs in the IDE. They have been formatted using the built in formatter and hence are not identical in layout to the programs in the book. This is to make copy and pasting them easier. The programs in the book are formatted to be easy to read on the page. Some of the programs are presented complete even though they were presented in the book as fragments.
If anything you consider important is missing or if you have any requests or comments contact:
This email address is being protected from spambots. You need JavaScript enabled to view it.
Page 50
#include <stdio.h>
#include "driver/gpio.h"
#include "freertos/FreeRTOS.h"
void app_main(void)
{
gpio_reset_pin(2);
gpio_set_direction(2, GPIO_MODE_OUTPUT);
while (1) {
gpio_set_level(2, 0);
vTaskDelay(1000 / portTICK_PERIOD_MS);
gpio_set_level(2, 1);
vTaskDelay(1000 / portTICK_PERIOD_MS);
}
}
Page 53
#include <stdio.h>
#include "driver/gpio.h"
#include "freertos/FreeRTOS.h"
void gpio_toggle(gpio_num_t gpio_num)
{
gpio_set_level(gpio_num, !gpio_get_level(gpio_num));
}
void app_main(void)
{
gpio_reset_pin(2);
gpio_set_direction(2, GPIO_MODE_INPUT_OUTPUT);
while (1)
{
gpio_toggle(2);
vTaskDelay(1000 / portTICK_PERIOD_MS);
}
}
Page 56
#include <stdio.h>
#include "driver/gpio.h"
#include "freertos/FreeRTOS.h"
void app_main(void)
{
gpio_reset_pin(2);
gpio_set_direction(2, GPIO_MODE_OUTPUT);
while (1)
{
gpio_set_level(2, 0);
gpio_set_level(2, 1);
}
}
Page 57
#include <stdio.h>
#include "driver/gpio.h"
#include "freertos/FreeRTOS.h"
void app_main(void)
{
gpio_reset_pin(2);
gpio_set_direction(2, GPIO_MODE_OUTPUT);
volatile int waste = 0;
while (1) {
gpio_set_level(2, 0);
waste++;
gpio_set_level(2, 1);
}
}
Page 59
#include <stdio.h>
#include "driver/gpio.h"
#include "freertos/FreeRTOS.h"
#include "esp_rom_sys.h"
#include <unistd.h>
void delay_us(int t)
{
usleep(t);
}
void app_main(void)
{
gpio_reset_pin(2);
gpio_set_direction(2, GPIO_MODE_OUTPUT);
while (1)
{
gpio_set_level(2, 0);
delay_us(1);
gpio_set_level(2, 1);
delay_us(1);
}
}
Page 59
#include "driver/gpio.h"
#include "freertos/FreeRTOS.h"
void app_main(void)
{
gpio_reset_pin(2);
gpio_set_direction(2, GPIO_MODE_OUTPUT);
volatile int i=0;
int n=1;
while (1) {
gpio_set_level(2, 0);
for(i=0;i<n;i++){}
gpio_set_level(2, 1);
for(i=0;i<n;i++){}
}
}
Page 62
#include <stdio.h>
#include "driver/gpio.h"
#include "freertos/FreeRTOS.h"
#include "esp_rom_sys.h"
#include <sys/time.h>
int64_t tick_us(int64_t offset)
{
static struct timeval tv_now;
gettimeofday(&tv_now, NULL);
return (int64_t)tv_now.tv_sec * 1000000L + (int64_t)tv_now.tv_usec + offset;
}
void delay_until(int64_t t)
{
static struct timeval tv_now;
do
{
gettimeofday(&tv_now, NULL);
} while (t > (int64_t)tv_now.tv_sec * 1000000L + (int64_t)tv_now.tv_usec);
}
void app_main(void)
{
int pin = 2;
gpio_reset_pin(pin);
gpio_set_direction(pin, GPIO_MODE_OUTPUT);
volatile int i = 0;
int n = 100;
int64_t t = 0;
int64_t width = 1000;
while (1)
{
t = tick_us(width);
gpio_set_level(pin, 1);
for (i = 0; i < n; i++)
{
}
delay_until(t);
t = tick_us(width);
gpio_set_level(pin, 0);
for (i = 0; i < n; i++)
{
}
delay_until(t);
}
}
Page 64
#include <stdio.h>
#include "driver/gpio.h"
#include "freertos/FreeRTOS.h"
#include "esp_rom_sys.h"
void app_main(void)
{
gpio_reset_pin(2);
gpio_set_direction(2, GPIO_MODE_OUTPUT);
gpio_reset_pin(4);
gpio_set_direction(4, GPIO_MODE_OUTPUT);
while (1) {
gpio_set_level(2, 1);
gpio_set_level(4, 0);
gpio_set_level(2, 0);
gpio_set_level(4, 1);
}
}
Page 66
#include <stdio.h>
#include "driver/gpio.h"
#include "freertos/FreeRTOS.h"
#include "esp_rom_sys.h"
#include "soc/gpio_reg.h"
void gpio_set(int32_t value, int32_t mask)
{
int32_t *OutAdd = (int32_t *)GPIO_OUT_REG;
*OutAdd = (*OutAdd & ~mask) | (value & mask);
}
void app_main(void)
{
gpio_reset_pin(2);
gpio_set_direction(2, GPIO_MODE_OUTPUT);
gpio_reset_pin(4);
gpio_set_direction(4, GPIO_MODE_OUTPUT);
int32_t mask = 1 << 2 | 1 << 4;
int32_t value1 = 0 << 2 | 1 << 4;
int32_t value2 = 1 << 2 | 0 << 4;
while (1)
{
gpio_set(value1, mask);
gpio_set(value2, mask);
}
}
Page 92
#include <stdio.h>
#include "driver/gpio.h"
#include "freertos/FreeRTOS.h"
void delay_ms(int t)
{
vTaskDelay(t / portTICK_PERIOD_MS);
}
void app_main(void)
{
gpio_reset_pin(2);
gpio_set_direction(2, GPIO_MODE_OUTPUT);
gpio_reset_pin(4);
gpio_set_direction(4, GPIO_MODE_INPUT);
gpio_pullup_en(4);
while (1)
{
if (gpio_get_level(4))
{
gpio_set_level(2, 1);
}
else
{
gpio_set_level(2, 0);
delay_ms(500);
}
}
}
Page 94
#include <stdio.h>
#include "driver/gpio.h"
#include "freertos/FreeRTOS.h"
void delay_ms(int t)
{
vTaskDelay(t / portTICK_PERIOD_MS);
}
void app_main(void)
{
gpio_reset_pin(2);
gpio_set_direction(2, GPIO_MODE_OUTPUT);
gpio_reset_pin(4);
gpio_set_direction(4, GPIO_MODE_INPUT);
gpio_pulldown_en(4);
while (1)
{
while (gpio_get_level(4) == 0)
{
};
delay_ms(10);
while (gpio_get_level(4) == 1)
{
};
gpio_set_level(2, 1);
delay_ms(1000);
gpio_set_level(2, 0);
}
}
Page 95
#include <stdio.h>
#include "driver/gpio.h"
#include "freertos/FreeRTOS.h"
#include <sys/time.h>
void delay_ms(int t)
{
vTaskDelay(t / portTICK_PERIOD_MS);
}
int64_t tick_ms(int64_t offset)
{
static struct timeval tv_now;
gettimeofday(&tv_now, NULL);
return (int64_t)tv_now.tv_sec * 1000L +
(int64_t)tv_now.tv_usec / 1000L + offset;
}
void app_main(void)
{
gpio_reset_pin(2);
gpio_set_direction(2, GPIO_MODE_OUTPUT);
gpio_reset_pin(4);
gpio_set_direction(4, GPIO_MODE_INPUT);
gpio_pulldown_en(4);
gpio_set_level(2, 0);
uint64_t t;
while (1)
{
while (gpio_get_level(4) == 0)
{
};
t = tick_ms(2000);
delay_ms(10);
while (gpio_get_level(4) == 1)
{
}
if (tick_ms(0) < t)
{
gpio_set_level(2, 1);
delay_ms(1000);
gpio_set_level(2, 0);
}
else
{
for (int i = 0; i < 10; i++)
{
gpio_set_level(2, 1);
delay_ms(1000);
gpio_set_level(2, 0);
delay_ms(1000);
}
}
}
}
Page 97
#include <stdio.h>
#include "driver/gpio.h"
#include "freertos/FreeRTOS.h"
#include <sys/time.h>
int64_t tick_us(int64_t offset) {
static struct timeval tv_now;
gettimeofday(&tv_now, NULL);
return (int64_t)tv_now.tv_sec * 1000000L +
(int64_t)tv_now.tv_usec + offset;
}
void delay_ms(int t) {
vTaskDelay(t / portTICK_PERIOD_MS);
}
void app_main(void)
{
gpio_reset_pin(4);
gpio_set_direction(4, GPIO_MODE_INPUT);
uint64_t t;
while (1) {
while (gpio_get_level(4) == 1) {};
while (gpio_get_level(4) == 0) {};
t = tick_us(0);
while (gpio_get_level(4) == 1) {};
t = tick_us(0) - t;
printf("%llu\n", t);
fflush(stdout);
delay_ms(1000);
}
}
Page 98
#include <stdio.h>
#include "driver/gpio.h"
#include "freertos/FreeRTOS.h"
#include <sys/time.h>
int64_t tick_us(int64_t offset)
{
static struct timeval tv_now;
gettimeofday(&tv_now, NULL);
return (int64_t)tv_now.tv_sec * 1000000L +
(int64_t)tv_now.tv_usec + offset;
}
void delay_ms(int t)
{
vTaskDelay(t / portTICK_PERIOD_MS);
}
void app_main(void)
{
gpio_reset_pin(4);
gpio_set_direction(4, GPIO_MODE_INPUT);
uint64_t t;
while (1)
{
while (gpio_get_level(4) == 1)
{
};
while (gpio_get_level(4) == 0)
{
};
t = 0;
while (gpio_get_level(4) == 1)
{
t++;
};
printf("%llu\n", t);
fflush(stdout);
delay_ms(1000);
}
Page 101
#include <stdio.h>
#include "driver/gpio.h"
#include "freertos/FreeRTOS.h"
#include <sys/time.h>
int64_t tick_us(int64_t offset)
{
static struct timeval tv_now;
gettimeofday(&tv_now, NULL);
return (int64_t)tv_now.tv_sec * 1000000L +
(int64_t)tv_now.tv_usec + offset;
}
void delay_until(int64_t t)
{
static struct timeval tv_now;
do
{
gettimeofday(&tv_now, NULL);
} while (t > (int64_t)tv_now.tv_sec * 1000000L +
(int64_t)tv_now.tv_usec);
}
void app_main(void)
{
gpio_reset_pin(4);
gpio_set_direction(4, GPIO_MODE_INPUT);
gpio_pulldown_en(4);
uint64_t t;
int s = 0;
int i;
int count = 0;
while (true)
{
i = gpio_get_level(4);
t = tick_us(100 * 1000);
switch (s)
{
case 0: // button not pushed
if (i)
{
s = 1;
count++;
printf("Button Push %d \n\r", count);
}
break;
case 1: // Button pushed
if (!i)
{
s = 0;
}
break;
default:
s = 0;
}
delay_until(t);
}
}
Page 103
#include <stdio.h>
#include "driver/gpio.h"
#include "freertos/FreeRTOS.h"
#include <sys/time.h>
#include <unistd.h>
void delay_us(int t)
{
usleep(t);
}
int64_t tick_us(int64_t offset)
{
static struct timeval tv_now;
gettimeofday(&tv_now, NULL);
return (int64_t)tv_now.tv_sec * 1000000L + (int64_t)tv_now.tv_usec + offset;
}
void delay_until(int64_t t)
{
static struct timeval tv_now;
do
{
gettimeofday(&tv_now, NULL);
} while (t > (int64_t)tv_now.tv_sec * 1000000L + (int64_t)tv_now.tv_usec);
}
void app_main(void)
{
gpio_reset_pin(4);
gpio_set_direction(4, GPIO_MODE_INPUT);
gpio_pulldown_en(4);
uint64_t t;
uint64_t tpush, twait;
int s = 0, i;
while (true)
{
i = gpio_get_level(4);
t = tick_us(0);
switch (s)
{
case 0: // button not pushed
if (i)
{
s = 1;
tpush = t;
}
break;
case 1: // Button pushed
if (!i)
{
s = 0;
if ((t - tpush) > 2000000)
{
printf("Button held \n\r");
}
else
{
printf("Button pushed \n\r");
}
fflush(stdout);
}
break;
default:
s = 0;
}
delay_until(t + 100 * 10000);
}
}
Page 105
#include <stdio.h>
#include "driver/gpio.h"
#include "freertos/FreeRTOS.h"
#include <sys/time.h>
int64_t tick_us(int64_t offset)
{
static struct timeval tv_now;
gettimeofday(&tv_now, NULL);
return (int64_t)tv_now.tv_sec * 1000000L + (int64_t)tv_now.tv_usec + offset;
}
void delay_until(int64_t t)
{
static struct timeval tv_now;
do
{
gettimeofday(&tv_now, NULL);
} while (t > (int64_t)tv_now.tv_sec * 1000000L + (int64_t)tv_now.tv_usec);
}
void app_main(void)
{
gpio_reset_pin(4);
gpio_set_direction(4, GPIO_MODE_INPUT);
gpio_pulldown_en(4);
gpio_reset_pin(2);
gpio_set_direction(2, GPIO_MODE_OUTPUT);
gpio_reset_pin(16);
gpio_set_direction(16, GPIO_MODE_OUTPUT);
gpio_reset_pin(17);
gpio_set_direction(17, GPIO_MODE_OUTPUT);
uint64_t t;
int edge;
int buttonNow;
int s = 0;
int buttonState = gpio_get_level(4);
gpio_set_level(2, 0);
gpio_set_level(16, 1);
gpio_set_level(17, 0);
while (true)
{
t = tick_us(0);
buttonNow = gpio_get_level(4);
edge = buttonState - buttonNow;
buttonState = buttonNow;
switch (s)
{
case 0:
if (edge == 1)
{
s = 1;
gpio_set_level(2, 0);
gpio_set_level(16, 1);
gpio_set_level(17, 0);
}
break;
case 1:
if (edge == 1)
{
s = 2;
gpio_set_level(2, 0);
gpio_set_level(16, 0);
gpio_set_level(17, 1);
}
break;
case 2:
if (edge == 1)
{
s = 0;
gpio_set_level(2, 1);
gpio_set_level(16, 0);
gpio_set_level(17, 0);
}
break;
default:
s = 0;
}
delay_until(t + 100 * 10000);
}
}
Page 113
#include <stdio.h>
#include "driver/gpio.h"
#include "freertos/FreeRTOS.h"
void ISR(void *arg)
{
gpio_intr_disable(4);
gpio_set_level(2, !gpio_get_level(2));
gpio_intr_enable(4);
}
void app_main(void)
{
gpio_isr_register(ISR, NULL, ESP_INTR_FLAG_LOWMED, NULL);
gpio_reset_pin(2);
gpio_set_direction(2, GPIO_MODE_INPUT_OUTPUT);
gpio_reset_pin(4);
gpio_set_direction(4, GPIO_MODE_INPUT);
gpio_intr_disable(4);
gpio_set_intr_type(4, GPIO_INTR_ANYEDGE);
gpio_intr_enable(4);
}
Page 114
#include <stdio.h>
#include "driver/gpio.h"
#include "freertos/FreeRTOS.h"
void ISR(void *arg)
{
gpio_intr_disable(16);
gpio_intr_disable(4);
gpio_set_level(2, !gpio_get_level(2));
gpio_intr_enable(16);
gpio_intr_enable(4);
}
void app_main(void)
{
gpio_isr_register(ISR, NULL, ESP_INTR_FLAG_LOWMED | ESP_INTR_FLAG_EDGE, NULL);
gpio_reset_pin(2);
gpio_set_direction(2, GPIO_MODE_INPUT_OUTPUT);
gpio_reset_pin(4);
gpio_set_direction(4, GPIO_MODE_INPUT);
gpio_intr_disable(4);
gpio_set_intr_type(4, GPIO_INTR_ANYEDGE);
gpio_reset_pin(16);
gpio_set_direction(16, GPIO_MODE_INPUT);
gpio_intr_disable(16);
gpio_set_intr_type(16, GPIO_INTR_ANYEDGE);
gpio_intr_enable(4);
gpio_intr_enable(16);
}
Page 115
#include <stdio.h>
#include "driver/gpio.h"
#include "freertos/FreeRTOS.h"
#include "soc/gpio_reg.h"
int32_t getIRQStatus()
{
return *(int *)GPIO_STATUS_REG;
}
void ISR(void *arg)
{
int32_t intStatus = getIRQStatus();
gpio_intr_disable(16);
gpio_intr_disable(4);
int32_t mask = 1 << 16;
if (intStatus & mask)
gpio_set_level(2, !gpio_get_level(2));
gpio_intr_enable(16);
gpio_intr_enable(4);
}
void app_main(void)
{
gpio_isr_register(ISR, NULL, ESP_INTR_FLAG_LOWMED | ESP_INTR_FLAG_EDGE, NULL);
gpio_reset_pin(2);
gpio_set_direction(2, GPIO_MODE_INPUT_OUTPUT);
gpio_reset_pin(4);
gpio_set_direction(4, GPIO_MODE_INPUT);
gpio_intr_disable(4);
gpio_set_intr_type(4, GPIO_INTR_ANYEDGE);
gpio_reset_pin(16);
gpio_set_direction(16, GPIO_MODE_INPUT);
gpio_intr_disable(16);
gpio_set_intr_type(16, GPIO_INTR_ANYEDGE);
gpio_intr_enable(4);
gpio_intr_enable(16);
}
Page 118
#include <stdio.h>
#include "driver/gpio.h"
#include "freertos/FreeRTOS.h"
#include <sys/time.h>
int64_t tick_us(int64_t offset)
{
static struct timeval tv_now;
gettimeofday(&tv_now, NULL);
return (int64_t)tv_now.tv_sec * 1000000L +
(int64_t)tv_now.tv_usec + offset;
}
void delay_ms(int t) {
vTaskDelay(t / portTICK_PERIOD_MS);
}
int64_t t = 0;
int state = 0;
void ISR(void *arg)
{
gpio_intr_disable(4);
t = tick_us(-t);
state++;
gpio_set_level(2, !gpio_get_level(2));
gpio_intr_enable(4);
}
void app_main(void)
{
gpio_isr_register(ISR, NULL, ESP_INTR_FLAG_LOWMED, NULL);
gpio_reset_pin(2);
gpio_set_direction(2, GPIO_MODE_INPUT_OUTPUT);
gpio_reset_pin(4);
gpio_set_direction(4, GPIO_MODE_INPUT);
gpio_intr_disable(4);
gpio_set_intr_type(4, GPIO_INTR_ANYEDGE);
gpio_intr_enable(4);
while (true)
{
while (state != 2)
{
};
gpio_intr_disable(4);
printf("time = %lld, %d\n", t, state);
fflush(stdout);
state = 0;
t = 0;
delay_ms(1000);
gpio_intr_enable(4);
}
}
Page 120
#include <stdio.h>
#include "driver/gpio.h"
#include "freertos/FreeRTOS.h"
char data[3];
void ISR(void *arg)
{
gpio_intr_disable(4);
for (int i = 0; i < 3; i++)
data[i] = 0;
gpio_intr_enable(4);
}
void app_main(void)
{
gpio_isr_register(ISR, NULL, ESP_INTR_FLAG_LOWMED, NULL);
gpio_reset_pin(4);
gpio_set_direction(4, GPIO_MODE_INPUT);
gpio_intr_disable(4);
gpio_set_intr_type(4, GPIO_INTR_ANYEDGE);
gpio_intr_enable(4);
while (true)
{
for (int i = 0; i < 3; i++)
data[i] = 0xFF;
if (data[0] != data[1] || data[1] != data[2] || data[2] != data[0])
break;
}
printf("%d %d %d\n", data[0], data[1], data[2]);
}
Page 122
#include <stdio.h>
#include "driver/gpio.h"
#include "freertos/FreeRTOS.h"
#include <sys/time.h>
int64_t tick_us(int64_t offset)
{
static struct timeval tv_now;
gettimeofday(&tv_now, NULL);
return (int64_t)tv_now.tv_sec * 1000000L +
(int64_t)tv_now.tv_usec + offset;
}
int64_t t = 0;
static QueueHandle_t gpio_evt_queue = NULL;
void ISR(void *arg)
{
gpio_intr_disable(4);
t = tick_us(0);
xQueueSendToBackFromISR(gpio_evt_queue, &t, NULL);
gpio_intr_enable(4);
}
void app_main(void)
{
gpio_evt_queue = xQueueCreate(10, sizeof(int32_t));
gpio_isr_register(ISR, NULL, ESP_INTR_FLAG_LOWMED, NULL);
gpio_reset_pin(4);
gpio_set_direction(4, GPIO_MODE_INPUT);
gpio_intr_disable(4);
gpio_set_intr_type(4, GPIO_INTR_ANYEDGE);
gpio_intr_enable(4);
int64_t time;
while (true)
{
if (xQueueReceive(gpio_evt_queue, &time, portMAX_DELAY))
{
printf("time = %lld\n", time);
fflush(stdout);
}
}
}
Page 125
#include <stdio.h>
#include "driver/gpio.h"
#include "freertos/FreeRTOS.h"
#include "driver/gptimer.h"
void app_main(void)
{
gptimer_config_t timer_config = {
.clk_src = GPTIMER_CLK_SRC_DEFAULT,
.direction = GPTIMER_COUNT_UP,
.resolution_hz = 1221,
};
gptimer_handle_t gptimer = NULL;
gptimer_new_timer(&timer_config, &gptimer);
gptimer_enable(gptimer);
gptimer_start(gptimer);
uint64_t count = 0;
do
{
gptimer_get_raw_count(gptimer, &count);
} while (count < 1000);
printf("Time up %lld\n", count);
gptimer_stop(gptimer);
gptimer_disable(gptimer);
gptimer_del_timer(gptimer);
}
Page 126
#include <stdio.h>
#include "driver/gpio.h"
#include "freertos/FreeRTOS.h"
#include "driver/gptimer.h"
gptimer_handle_t tick_us_start(void)
{
gptimer_config_t timer_config = {
.clk_src = GPTIMER_CLK_SRC_DEFAULT,
.direction = GPTIMER_COUNT_UP,
.resolution_hz = 1000000,
};
gptimer_handle_t gptimer_us = NULL;
gptimer_new_timer(&timer_config, &gptimer_us);
gptimer_enable(gptimer_us);
gptimer_start(gptimer_us);
return gptimer_us;
}
int64_t tick_us(gptimer_handle_t gptimer_us, int64_t offset)
{
uint64_t count;
gptimer_get_raw_count(gptimer_us, &count);
return count + offset;
}
void app_main(void)
{
gptimer_handle_t gptimer_us = tick_us_start();
int64_t tick = tick_us(gptimer_us, 1000000);
while (tick_us(gptimer_us, 0) < tick)
{
};
printf("time up %lld\n", tick_us(gptimer_us, 0));
}
Page 127
#include <stdio.h>
#include "driver/gpio.h"
#include "freertos/FreeRTOS.h"
#include "driver/gptimer.h"
bool ISR(gptimer_handle_t gptimer,
const gptimer_alarm_event_data_t *edata,
void *user_data)
{
gpio_set_level(2, !gpio_get_level(2));
return true;
}
void app_main(void)
{
gpio_set_direction(2, GPIO_MODE_INPUT_OUTPUT);
gpio_reset_pin(2);
gpio_set_direction(2, GPIO_MODE_INPUT_OUTPUT);
gptimer_handle_t gptimer = NULL;
gptimer_config_t timer_config = {
.clk_src = GPTIMER_CLK_SRC_DEFAULT,
.direction = GPTIMER_COUNT_UP,
.resolution_hz = 1221,
};
gptimer_new_timer(&timer_config, &gptimer);
gptimer_alarm_config_t alarm_config = {
.flags.auto_reload_on_alarm = true,
.alarm_count = 1000,
.reload_count = 0};
gptimer_set_alarm_action(gptimer, &alarm_config);
gptimer_event_callbacks_t cbs = {
.on_alarm = ISR,
};
gptimer_register_event_callbacks(gptimer, &cbs, NULL);
gptimer_enable(gptimer);
gptimer_start(gptimer);
while (true)
{
};
}
Page 140
#include <stdio.h>
#include "driver/gpio.h"
#include "freertos/FreeRTOS.h"
#include "driver/ledc.h"
void app_main(void)
{
ledc_timer_config_t ledc_timer = {
.speed_mode = LEDC_LOW_SPEED_MODE,
.timer_num = 0,
.duty_resolution = LEDC_TIMER_13_BIT,
.freq_hz = 4000,
.clk_cfg = LEDC_APB_CLK};
ledc_timer_config(&ledc_timer);
ledc_channel_config_t ledc_channel = {
.speed_mode = LEDC_LOW_SPEED_MODE,
.channel = 0,
.timer_sel = 0,
.intr_type = LEDC_INTR_DISABLE,
.gpio_num = 2,
.duty = 4096,
.hpoint = 0};
ledc_channel_config(&ledc_channel);
}
Page 143
#include <stdio.h>
#include "driver/gpio.h"
#include "freertos/FreeRTOS.h"
#include "esp_rom_sys.h"
#include "driver/ledc.h"
#include <unistd.h>
void delay_us(int t)
{
usleep(t);
}
void PWMconfigLow(int gpio, int chan, int timer, int res,
int freq, float duty)
{
ledc_timer_config_t ledc_timer = {
.speed_mode = LEDC_LOW_SPEED_MODE,
.clk_cfg = LEDC_APB_CLK};
ledc_timer.timer_num = timer;
ledc_timer.duty_resolution = res;
ledc_timer.freq_hz = freq;
ledc_channel_config_t ledc_channel = {
.speed_mode = LEDC_LOW_SPEED_MODE,
.hpoint = 0,
.intr_type = LEDC_INTR_DISABLE,
};
ledc_channel.channel = chan;
ledc_channel.timer_sel = timer;
ledc_channel.gpio_num = gpio;
ledc_channel.duty = ((float)(2 << (res - 1))) * duty;
ledc_timer_config(&ledc_timer);
ledc_channel_config(&ledc_channel);
}
void changeDutyLow(int chan, int res, float duty)
{
ledc_set_duty(LEDC_LOW_SPEED_MODE, chan,
((float)(2 << (res - 1))) * duty);
ledc_update_duty(LEDC_LOW_SPEED_MODE, chan);
}
void app_main(void)
{
PWMconfigLow(2, 0, 3, 13, 4000, 0.25);
while (true)
{
delay_us(1000);
changeDutyLow(0, 13, 0.5);
delay_us(1000);
changeDutyLow(0, 13, 0.25);
}
}
Page 146
#include <stdio.h>
#include "freertos/FreeRTOS.h"
#include "esp_rom_sys.h"
#include <sys/time.h>
#include "driver/ledc.h"
#include "math.h"
#include <unistd.h>
void delay_us(int t)
{
usleep(t);
}
void PWMconfigLow(int gpio, int chan, int timer, int res, int freq, float duty)
{
ledc_timer_config_t ledc_timer = {
.speed_mode = LEDC_LOW_SPEED_MODE,
.clk_cfg = LEDC_APB_CLK};
ledc_timer.timer_num = timer;
ledc_timer.duty_resolution = res;
ledc_timer.freq_hz = freq;
ledc_channel_config_t ledc_channel = {
.speed_mode = LEDC_LOW_SPEED_MODE,
.hpoint = 0,
.intr_type = LEDC_INTR_DISABLE,
};
ledc_channel.channel = chan;
ledc_channel.timer_sel = timer;
ledc_channel.gpio_num = gpio;
ledc_channel.duty = ((float)(2 << (res - 1))) * duty;
ledc_timer_config(&ledc_timer);
ledc_channel_config(&ledc_channel);
}
uint8_t wave[256];
void app_main(void)
{
for (int i = 0; i < 256; i++)
{
wave[i] = (uint8_t)((128.0 +
sinf((float)i * 2.0 * 3.14159 / 255.0) * 128.0));
}
int f = 60000;
int t = (1 * 1000 * 1000) / f;
PWMconfigLow(2, 0, 0, 8, f, 0.25);
fflush(stdout);
while (true)
{
for (int i = 0; i < 256; i++)
{
ledc_set_duty(LEDC_LOW_SPEED_MODE, 0, wave[i]);
ledc_update_duty(LEDC_LOW_SPEED_MODE, 0);
delay_us(t);
}
}
}
Page 148
#include <stdio.h>
#include "driver/gpio.h"
#include "freertos/FreeRTOS.h"
#include "esp_rom_sys.h"
#include <sys/time.h>
#include "driver/ledc.h"
void delay_ms(int t)
{
vTaskDelay(t / portTICK_PERIOD_MS);
}
void PWMconfigLow(int gpio, int chan, int timer, int res, int freq, float duty)
{
ledc_timer_config_t ledc_timer = {
.speed_mode = LEDC_LOW_SPEED_MODE,
.clk_cfg = LEDC_APB_CLK};
ledc_timer.timer_num = timer;
ledc_timer.duty_resolution = res;
ledc_timer.freq_hz = freq;
ledc_channel_config_t ledc_channel = {
.speed_mode = LEDC_LOW_SPEED_MODE,
.hpoint = 0,
.intr_type = LEDC_INTR_DISABLE,
};
ledc_channel.channel = chan;
ledc_channel.timer_sel = timer;
ledc_channel.gpio_num = gpio;
ledc_channel.duty = ((float)(2 << (res - 1))) * duty;
ledc_timer_config(&ledc_timer);
ledc_channel_config(&ledc_channel);
}
void app_main(void)
{
while (true)
{
PWMconfigLow(2, 0, 0, 8, 2000, 0.5);
for (int d = 0; d <= 256; d++)
{
ledc_set_duty(LEDC_LOW_SPEED_MODE, 0, d);
ledc_update_duty(LEDC_LOW_SPEED_MODE, 0);
delay_ms(10);
}
}
}
Page 150 complete program to do cubic dimming
#include <stdio.h>
#include "driver/gpio.h"
#include "freertos/FreeRTOS.h"
#include "esp_rom_sys.h"
#include <sys/time.h>
#include "driver/ledc.h"
void delay_ms(int t)
{
vTaskDelay(t / portTICK_PERIOD_MS);
}
void PWMconfigLow(int gpio, int chan, int timer, int res, int freq, float duty)
{
ledc_timer_config_t ledc_timer = {
.speed_mode = LEDC_LOW_SPEED_MODE,
.clk_cfg = LEDC_APB_CLK};
ledc_timer.timer_num = timer;
ledc_timer.duty_resolution = res;
ledc_timer.freq_hz = freq;
ledc_channel_config_t ledc_channel = {
.speed_mode = LEDC_LOW_SPEED_MODE,
.hpoint = 0,
.intr_type = LEDC_INTR_DISABLE,
};
ledc_channel.channel = chan;
ledc_channel.timer_sel = timer;
ledc_channel.gpio_num = gpio;
ledc_channel.duty = ((float)(2 << (res - 1))) * duty;
ledc_timer_config(&ledc_timer);
ledc_channel_config(&ledc_channel);
}
void app_main(void)
{
while (true)
{
PWMconfigLow(2, 0, 0, 8, 2000, 0.5);
for (int d = 0; d <= 256; d++)
{
ledc_set_duty(LEDC_LOW_SPEED_MODE, 0, d * d * d / 255 / 255);
ledc_update_duty(LEDC_LOW_SPEED_MODE, 0);
delay_ms(10);
}
}
}
Page 154
#include <stdio.h>
#include "freertos/FreeRTOS.h"
#include "driver/ledc.h"
void PWMconfigLow(int gpio, int chan, int timer, int res, int freq, float duty)
{
ledc_timer_config_t ledc_timer = {
.speed_mode = LEDC_LOW_SPEED_MODE,
.clk_cfg = LEDC_APB_CLK};
ledc_timer.timer_num = timer;
ledc_timer.duty_resolution = res;
ledc_timer.freq_hz = freq;
ledc_channel_config_t ledc_channel = {
.speed_mode = LEDC_LOW_SPEED_MODE,
.hpoint = 0,
.intr_type = LEDC_INTR_DISABLE,
};
ledc_channel.channel = chan;
ledc_channel.timer_sel = timer;
ledc_channel.gpio_num = gpio;
ledc_channel.duty = ((float)(2 << (res - 1))) * duty;
ledc_timer.deconfigure = false;
ledc_timer_config(&ledc_timer);
ledc_channel_config(&ledc_channel);
}
void app_main(void)
{
PWMconfigLow(2, 0, 0, 8, 2550, 0);
ledc_fade_func_install(LEDC_INTR_DISABLE);
while (true)
{
ledc_set_fade_with_time(LEDC_LOW_SPEED_MODE, 0, 255, 1000);
ledc_fade_start(LEDC_LOW_SPEED_MODE, 0, LEDC_FADE_WAIT_DONE);
ledc_set_fade_with_time(LEDC_LOW_SPEED_MODE, 0, 0, 1000);
ledc_fade_start(LEDC_LOW_SPEED_MODE, 0, LEDC_FADE_WAIT_DONE);
}
}
Page 155 complete program from fragment
#include <stdio.h>
#include "freertos/FreeRTOS.h"
#include "driver/ledc.h"
void PWMconfigLow(int gpio, int chan, int timer, int res, int freq, float duty)
{
ledc_timer_config_t ledc_timer = {
.speed_mode = LEDC_LOW_SPEED_MODE,
.clk_cfg = LEDC_APB_CLK};
ledc_timer.timer_num = timer;
ledc_timer.duty_resolution = res;
ledc_timer.freq_hz = freq;
ledc_channel_config_t ledc_channel = {
.speed_mode = LEDC_LOW_SPEED_MODE,
.hpoint = 0,
.intr_type = LEDC_INTR_DISABLE,
};
ledc_channel.channel = chan;
ledc_channel.timer_sel = timer;
ledc_channel.gpio_num = gpio;
ledc_channel.duty = ((float)(2 << (res - 1))) * duty;
ledc_timer.deconfigure = false;
ledc_timer_config(&ledc_timer);
ledc_channel_config(&ledc_channel);
}
void app_main(void)
{
PWMconfigLow(2, 0, 0, 8, 2550, 0);
ledc_fade_func_install(LEDC_INTR_DISABLE);
while (true)
{
ledc_set_fade_with_step(LEDC_LOW_SPEED_MODE, 0, 255, 1, 10);
ledc_fade_start(LEDC_LOW_SPEED_MODE, 0, LEDC_FADE_WAIT_DONE);
ledc_set_fade_with_step(LEDC_LOW_SPEED_MODE, 0, 0, 1, 10);
ledc_fade_start(LEDC_LOW_SPEED_MODE, 0, LEDC_FADE_WAIT_DONE);
}
}
Page 156
#include <stdio.h>
#include "freertos/FreeRTOS.h"
#include "driver/ledc.h"
void PWMconfigLow(int gpio, int chan, int timer, int res, int freq, float duty)
{
ledc_timer_config_t ledc_timer = {
.speed_mode = LEDC_LOW_SPEED_MODE,
.clk_cfg = LEDC_APB_CLK};
ledc_timer.timer_num = timer;
ledc_timer.duty_resolution = res;
ledc_timer.freq_hz = freq;
ledc_channel_config_t ledc_channel = {
.speed_mode = LEDC_LOW_SPEED_MODE,
.hpoint = 0,
.intr_type = LEDC_INTR_DISABLE,
};
ledc_channel.channel = chan;
ledc_channel.timer_sel = timer;
ledc_channel.gpio_num = gpio;
ledc_channel.duty = ((float)(2 << (res - 1))) * duty;
ledc_timer.deconfigure = false;
ledc_timer_config(&ledc_timer);
ledc_channel_config(&ledc_channel);
}
void changeDutyPhaseLow(int chan, int res, float duty, float phase)
{
ledc_set_duty_with_hpoint(LEDC_LOW_SPEED_MODE, chan,
((float)(2 << (res - 1))) * duty,
((float)(2 << (res - 1))) * phase);
ledc_update_duty(LEDC_LOW_SPEED_MODE, chan);
}
void app_main(void)
{
PWMconfigLow(2, 0, 0, 8, 2550, 0.5);
PWMconfigLow(4, 1, 0, 8, 2550, 0.5);
changeDutyPhaseLow(1, 8, 0.5, 0.25);
}
Page 165
#include <stdio.h>
#include "freertos/FreeRTOS.h"
#include "driver/mcpwm_prelude.h"
void app_main(void)
{
mcpwm_timer_handle_t timer = NULL;
mcpwm_timer_config_t timer_config = {
.group_id = 0,
.clk_src = MCPWM_TIMER_CLK_SRC_DEFAULT,
.resolution_hz = 1000000,
.period_ticks = 20000,
.count_mode = MCPWM_TIMER_COUNT_MODE_UP,
};
mcpwm_new_timer(&timer_config, &timer);
mcpwm_oper_handle_t oper = NULL;
mcpwm_operator_config_t operator_config = {
.group_id = 0,
};
mcpwm_new_operator(&operator_config, &oper);
mcpwm_operator_connect_timer(oper, timer);
mcpwm_gen_handle_t generator = NULL;
mcpwm_generator_config_t generator_config = {
.gen_gpio_num = 2,
};
mcpwm_new_generator(oper, &generator_config, &generator);
mcpwm_generator_set_action_on_timer_event(generator,
MCPWM_GEN_TIMER_EVENT_ACTION(MCPWM_TIMER_DIRECTION_UP,
MCPWM_TIMER_EVENT_FULL, MCPWM_GEN_ACTION_TOGGLE));
mcpwm_timer_enable(timer);
mcpwm_timer_start_stop(timer, MCPWM_TIMER_START_NO_STOP);
}
Page 167 complete program from fragment
#include <stdio.h>
#include "freertos/FreeRTOS.h"
#include "driver/mcpwm_prelude.h"
void app_main(void)
{
mcpwm_timer_handle_t timer = NULL;
mcpwm_timer_config_t timer_config = {
.group_id = 0,
.clk_src = MCPWM_TIMER_CLK_SRC_DEFAULT,
.resolution_hz = 1000000,
.period_ticks = 20000,
.count_mode = MCPWM_TIMER_COUNT_MODE_UP,
};
mcpwm_new_timer(&timer_config, &timer);
mcpwm_oper_handle_t oper = NULL;
mcpwm_operator_config_t operator_config = {
.group_id = 0,
};
mcpwm_new_operator(&operator_config, &oper);
mcpwm_operator_connect_timer(oper, timer);
mcpwm_gen_handle_t generator = NULL;
mcpwm_generator_config_t generator_config = {
.gen_gpio_num = 2,
};
mcpwm_new_generator(oper, &generator_config, &generator);
mcpwm_cmpr_handle_t comparator = NULL;
mcpwm_comparator_config_t comparator_config = {
.flags.update_cmp_on_tez = true,
};
mcpwm_new_comparator(oper, &comparator_config, &comparator);
mcpwm_comparator_set_compare_value(comparator,10000);
mcpwm_generator_set_action_on_timer_event(generator,
MCPWM_GEN_TIMER_EVENT_ACTION(MCPWM_TIMER_DIRECTION_UP,
MCPWM_TIMER_EVENT_EMPTY, MCPWM_GEN_ACTION_HIGH));
mcpwm_generator_set_action_on_compare_event(generator,
MCPWM_GEN_COMPARE_EVENT_ACTION(MCPWM_TIMER_DIRECTION_UP,
comparator, MCPWM_GEN_ACTION_LOW));
mcpwm_timer_enable(timer);
mcpwm_timer_start_stop(timer, MCPWM_TIMER_START_NO_STOP);
}
Page 169 complete program from fragment
#include <stdio.h>
#include "freertos/FreeRTOS.h"
#include "driver/mcpwm_prelude.h"
void app_main(void)
{
mcpwm_timer_handle_t timer = NULL;
mcpwm_timer_config_t timer_config = {
.group_id = 0,
.clk_src = MCPWM_TIMER_CLK_SRC_DEFAULT,
.resolution_hz = 1000000,
.period_ticks = 20000,
.count_mode = MCPWM_TIMER_COUNT_MODE_UP,
};
mcpwm_new_timer(&timer_config, &timer);
mcpwm_oper_handle_t oper = NULL;
mcpwm_operator_config_t operator_config = {
.group_id = 0,
};
mcpwm_new_operator(&operator_config, &oper);
mcpwm_operator_connect_timer(oper, timer);
mcpwm_generator_config_t generator_config = {
.gen_gpio_num = 2,
};
mcpwm_gen_handle_t generatorA = NULL;
mcpwm_new_generator(oper, &generator_config, &generatorA);
generator_config.gen_gpio_num = 4;
mcpwm_gen_handle_t generatorB = NULL;
mcpwm_new_generator(oper, &generator_config, &generatorB);
mcpwm_comparator_config_t comparator_config = {
.flags.update_cmp_on_tez = true,
};
mcpwm_cmpr_handle_t comparatorA = NULL;
mcpwm_new_comparator(oper, &comparator_config, &comparatorA);
mcpwm_cmpr_handle_t comparatorB = NULL;
mcpwm_new_comparator(oper, &comparator_config, &comparatorB);
mcpwm_comparator_set_compare_value(comparatorA, 10000);
mcpwm_comparator_set_compare_value(comparatorB, 5000);
mcpwm_generator_set_action_on_timer_event(generatorA,
MCPWM_GEN_TIMER_EVENT_ACTION(MCPWM_TIMER_DIRECTION_UP,
MCPWM_TIMER_EVENT_EMPTY, MCPWM_GEN_ACTION_HIGH));
mcpwm_generator_set_action_on_compare_event(generatorA,
MCPWM_GEN_COMPARE_EVENT_ACTION(MCPWM_TIMER_DIRECTION_UP,
comparatorA, MCPWM_GEN_ACTION_LOW));
mcpwm_generator_set_action_on_timer_event(generatorB,
MCPWM_GEN_TIMER_EVENT_ACTION(MCPWM_TIMER_DIRECTION_UP,
MCPWM_TIMER_EVENT_EMPTY, MCPWM_GEN_ACTION_HIGH));
mcpwm_generator_set_action_on_compare_event(generatorB,
MCPWM_GEN_COMPARE_EVENT_ACTION(MCPWM_TIMER_DIRECTION_UP,
comparatorB, MCPWM_GEN_ACTION_LOW));
mcpwm_timer_enable(timer);
mcpwm_timer_start_stop(timer, MCPWM_TIMER_START_NO_STOP);
}
Page 171 complete program from fragment
#include <stdio.h>
#include "freertos/FreeRTOS.h"
#include "driver/mcpwm_prelude.h"
void app_main(void)
{
mcpwm_timer_handle_t timer = NULL;
mcpwm_timer_config_t timer_config = {
.group_id = 0,
.clk_src = MCPWM_TIMER_CLK_SRC_DEFAULT,
.resolution_hz = 1000000,
.period_ticks = 20000,
.count_mode = MCPWM_TIMER_COUNT_MODE_UP_DOWN,
};
mcpwm_new_timer(&timer_config, &timer);
mcpwm_oper_handle_t oper = NULL;
mcpwm_operator_config_t operator_config = {
.group_id = 0,
};
mcpwm_new_operator(&operator_config, &oper);
mcpwm_operator_connect_timer(oper, timer);
mcpwm_generator_config_t generator_config = {
.gen_gpio_num = 2,
};
mcpwm_gen_handle_t generatorA = NULL;
mcpwm_new_generator(oper, &generator_config, &generatorA);
generator_config.gen_gpio_num = 4;
mcpwm_gen_handle_t generatorB = NULL;
mcpwm_new_generator(oper, &generator_config, &generatorB);
mcpwm_comparator_config_t comparator_config = {
.flags.update_cmp_on_tez = true,
};
mcpwm_cmpr_handle_t comparatorA = NULL;
mcpwm_new_comparator(oper, &comparator_config, &comparatorA);
mcpwm_cmpr_handle_t comparatorB = NULL;
mcpwm_new_comparator(oper, &comparator_config, &comparatorB);
mcpwm_comparator_set_compare_value(comparatorA, 10000);
mcpwm_comparator_set_compare_value(comparatorB, 5000);
mcpwm_comparator_set_compare_value(comparatorA, 5000);
mcpwm_comparator_set_compare_value(comparatorB, 9000);
mcpwm_generator_set_action_on_compare_event(generatorA,
MCPWM_GEN_COMPARE_EVENT_ACTION(MCPWM_TIMER_DIRECTION_UP,
comparatorA, MCPWM_GEN_ACTION_HIGH));
mcpwm_generator_set_action_on_compare_event(generatorA,
MCPWM_GEN_COMPARE_EVENT_ACTION(MCPWM_TIMER_DIRECTION_DOWN,
comparatorA, MCPWM_GEN_ACTION_LOW));
mcpwm_generator_set_action_on_compare_event(generatorB,
MCPWM_GEN_COMPARE_EVENT_ACTION(MCPWM_TIMER_DIRECTION_UP,
comparatorB, MCPWM_GEN_ACTION_HIGH));
mcpwm_generator_set_action_on_compare_event(generatorB,
MCPWM_GEN_COMPARE_EVENT_ACTION(MCPWM_TIMER_DIRECTION_DOWN,
comparatorB, MCPWM_GEN_ACTION_LOW));
mcpwm_timer_enable(timer);
mcpwm_timer_start_stop(timer, MCPWM_TIMER_START_NO_STOP);
}
Page 174 MCPWM.h
#include "driver/mcpwm_prelude.h"
mcpwm_timer_handle_t makeTimer(uint32_t res, uint32_t periodTicks)
{
mcpwm_timer_handle_t timer = NULL;
mcpwm_timer_config_t timer_config = {
.group_id = 0,
.clk_src = MCPWM_TIMER_CLK_SRC_DEFAULT,
.resolution_hz = res,
.period_ticks = periodTicks,
.count_mode = MCPWM_TIMER_COUNT_MODE_UP,
};
mcpwm_new_timer(&timer_config, &timer);
return timer;
}
mcpwm_oper_handle_t makeOper()
{
mcpwm_oper_handle_t oper = NULL;
mcpwm_operator_config_t operator_config = {
.group_id = 0,
};
mcpwm_new_operator(&operator_config, &oper);
return oper;
}
mcpwm_gen_handle_t makeGen(mcpwm_oper_handle_t oper, int gpio)
{
mcpwm_generator_config_t generator_config = {
.gen_gpio_num = gpio};
mcpwm_gen_handle_t generator = NULL;
mcpwm_new_generator(oper, &generator_config, &generator);
return generator;
}
mcpwm_cmpr_handle_t makeComp(mcpwm_oper_handle_t oper)
{
mcpwm_comparator_config_t comparator_config = {
.flags.update_cmp_on_tez = true,
};
mcpwm_cmpr_handle_t comparator = NULL;
mcpwm_new_comparator(oper, &comparator_config, &comparator);
return comparator;
}
void setPhaseTep(mcpwm_timer_handle_t SourceTimer, mcpwm_timer_handle_t PhasedTimer, uint32_t phaseticks)
{
mcpwm_timer_sync_src_config_t syncconfig = {
.timer_event = MCPWM_TIMER_EVENT_EMPTY,
};
mcpwm_sync_handle_t sync = NULL;
mcpwm_new_timer_sync_src(SourceTimer, &syncconfig, &sync);
mcpwm_timer_sync_phase_config_t phase = {
.count_value = phaseticks,
.direction = MCPWM_TIMER_DIRECTION_UP,
.sync_src = sync};
mcpwm_timer_set_phase_on_sync(PhasedTimer, &phase);
}
Page 176 Uses previous header
#include <stdio.h>
#include "freertos/FreeRTOS.h"
#include "driver/mcpwm_prelude.h"
#include "MCPWM.h"
void app_main(void)
{
mcpwm_timer_handle_t timer1 = makeTimer(1000000, 20000);
mcpwm_timer_handle_t timer2 = makeTimer(1000000, 20000);
mcpwm_oper_handle_t oper1 = makeOper();
mcpwm_oper_handle_t oper2 = makeOper();
mcpwm_operator_connect_timer(oper1, timer1);
mcpwm_operator_connect_timer(oper2, timer2);
mcpwm_gen_handle_t generator1 = makeGen(oper1, 2);
mcpwm_gen_handle_t generator2 = makeGen(oper2, 4);
mcpwm_cmpr_handle_t comparator1 = makeComp(oper1);
mcpwm_cmpr_handle_t comparator2 = makeComp(oper2);
mcpwm_comparator_set_compare_value(comparator1, 9000);
mcpwm_comparator_set_compare_value(comparator2, 9000);
setPhaseTep(timer1, timer2, 4000);
mcpwm_generator_set_action_on_timer_event(generator1,
MCPWM_GEN_TIMER_EVENT_ACTION(MCPWM_TIMER_DIRECTION_UP,
MCPWM_TIMER_EVENT_EMPTY, MCPWM_GEN_ACTION_HIGH));
mcpwm_generator_set_action_on_compare_event(generator1,
MCPWM_GEN_COMPARE_EVENT_ACTION(MCPWM_TIMER_DIRECTION_UP,
comparator1, MCPWM_GEN_ACTION_LOW));
mcpwm_generator_set_action_on_timer_event(generator2,
MCPWM_GEN_TIMER_EVENT_ACTION(MCPWM_TIMER_DIRECTION_UP,
MCPWM_TIMER_EVENT_EMPTY, MCPWM_GEN_ACTION_HIGH));
mcpwm_generator_set_action_on_compare_event(generator2,
MCPWM_GEN_COMPARE_EVENT_ACTION(MCPWM_TIMER_DIRECTION_UP,
comparator2, MCPWM_GEN_ACTION_LOW));
mcpwm_timer_enable(timer1);
mcpwm_timer_start_stop(timer1, MCPWM_TIMER_START_NO_STOP);
mcpwm_timer_enable(timer2);
mcpwm_timer_start_stop(timer2, MCPWM_TIMER_START_NO_STOP);
}
Page 184 -186 uses MCPWM.h given earlier
#include <stdio.h>
#include "freertos/FreeRTOS.h"
#include "driver/mcpwm_prelude.h"
#include "MCPWM.h"
typedef struct{
mcpwm_timer_handle_t timer;
mcpwm_oper_handle_t oper;
mcpwm_gen_handle_t generator1;
mcpwm_cmpr_handle_t comparator1;
}PWM;
PWM makePWM(int gpio, uint32_t res, uint32_t ticksperiod,
uint32_t duty) {
PWM pwm;
pwm.timer = makeTimer(res, ticksperiod);
pwm.oper = makeOper();
mcpwm_operator_connect_timer(pwm.oper, pwm.timer);
pwm.generator1 = makeGen(pwm.oper, gpio);
pwm.comparator1 = makeComp(pwm.oper);
mcpwm_comparator_set_compare_value(pwm.comparator1, duty);
mcpwm_generator_set_action_on_timer_event(pwm.generator1,
MCPWM_GEN_TIMER_EVENT_ACTION(MCPWM_TIMER_DIRECTION_UP,
MCPWM_TIMER_EVENT_EMPTY, MCPWM_GEN_ACTION_HIGH));
mcpwm_generator_set_action_on_compare_event(pwm.generator1,
MCPWM_GEN_COMPARE_EVENT_ACTION(MCPWM_TIMER_DIRECTION_UP,
pwm.comparator1, MCPWM_GEN_ACTION_LOW));
mcpwm_timer_enable(pwm.timer);
mcpwm_timer_start_stop(pwm.timer, MCPWM_TIMER_START_NO_STOP);
return pwm;
}
typedef struct
{
PWM pwm;
uint32_t gpio;
uint32_t duty;
uint32_t freq;
uint32_t resolution;
float speed;
} Motor;
Motor makeUniMotor(int32_t gpio) {
int32_t res = 1000000;
int32_t freq = 500;
Motor motor;
motor.gpio = gpio;
motor.pwm = makePWM(gpio, res, freq, 0);
motor.freq = freq;
motor.speed = 0;
motor.duty = 0;
return motor;
}
void setUniMotorSpeed(Motor motor, float speed) {
motor.speed = speed;
motor.duty = speed * (float)(motor.freq);
mcpwm_comparator_set_compare_value(motor.pwm.comparator1,
motor.duty);
}
void app_main(void)
{
Motor motor = makeUniMotor(2);
setUniMotorSpeed(motor, 0.1);
}
Page 191-192 uses MCPWM.h given earlier
#include <stdio.h>
#include "freertos/FreeRTOS.h"
#include "driver/mcpwm_prelude.h"
#include "MCPWM.h"
typedef struct{
mcpwm_timer_handle_t timer;
mcpwm_oper_handle_t oper;
mcpwm_gen_handle_t generator1;
mcpwm_cmpr_handle_t comparator1;
}PWM;
PWM makePWM(int gpio, uint32_t res, uint32_t ticksperiod,
uint32_t duty) {
PWM pwm;
pwm.timer = makeTimer(res, ticksperiod);
pwm.oper = makeOper();
mcpwm_operator_connect_timer(pwm.oper, pwm.timer);
pwm.generator1 = makeGen(pwm.oper, gpio);
pwm.comparator1 = makeComp(pwm.oper);
mcpwm_comparator_set_compare_value(pwm.comparator1, duty);
mcpwm_generator_set_action_on_timer_event(pwm.generator1,
MCPWM_GEN_TIMER_EVENT_ACTION(MCPWM_TIMER_DIRECTION_UP,
MCPWM_TIMER_EVENT_EMPTY, MCPWM_GEN_ACTION_HIGH));
mcpwm_generator_set_action_on_compare_event(pwm.generator1,
MCPWM_GEN_COMPARE_EVENT_ACTION(MCPWM_TIMER_DIRECTION_UP,
pwm.comparator1, MCPWM_GEN_ACTION_LOW));
mcpwm_timer_enable(pwm.timer);
mcpwm_timer_start_stop(pwm.timer, MCPWM_TIMER_START_NO_STOP);
return pwm;
}
typedef struct
{
PWM pwm;
PWM pwm2;
uint32_t gpio;
uint32_t gpio2;
uint32_t duty;
uint32_t freq;
uint32_t resolution;
float speed;
bool forward;
} Motor;
Motor makeBiMotor(int32_t gpio1,int32_t gpio2) {
int32_t res = 1000000;
int32_t freq = 500;
Motor motor;
motor.gpio = gpio1;
motor.gpio2 = gpio2;
motor.pwm = makePWM(gpio1, res, freq, 0);
motor.pwm2 = makePWM(gpio2, res, freq, 0);
motor.freq = freq;
motor.speed = 0;
motor.duty = 0;
motor.forward=true;
return motor;
}
void setBiMotorSpeedDirection(Motor motor, float speed,
bool forward) {
motor.speed = speed;
motor.duty = speed * (float)(motor.freq);
motor.forward=forward;
if(forward){
mcpwm_comparator_set_compare_value(motor.pwm2.comparator1,0);
mcpwm_comparator_set_compare_value(motor.pwm.comparator1,
motor.duty);
}else{
mcpwm_comparator_set_compare_value(motor.pwm.comparator1, 0);
mcpwm_comparator_set_compare_value(motor.pwm2.comparator1,
motor.duty);
}
}
void app_main(void)
{
Motor motor = makeBiMotor(2,4);
setBiMotorSpeedDirection(motor, 0.1,false);
}
Page 194 -195 uses MCPWM.h given earlier and only partially listed on page
#include <stdio.h>
#include "freertos/FreeRTOS.h"
#include "driver/mcpwm_prelude.h"
#include "MCPWM.h"
typedef struct
{
mcpwm_timer_handle_t timer;
mcpwm_oper_handle_t oper;
mcpwm_gen_handle_t generator1;
mcpwm_gen_handle_t generator2;
mcpwm_cmpr_handle_t comparator1;
} PWM;
PWM makePWM(int gpio, uint32_t res, uint32_t ticksperiod,
uint32_t duty)
{
PWM pwm;
pwm.timer = makeTimer(res, ticksperiod);
pwm.oper = makeOper();
mcpwm_operator_connect_timer(pwm.oper, pwm.timer);
pwm.generator1 = makeGen(pwm.oper, gpio);
pwm.comparator1 = makeComp(pwm.oper);
mcpwm_comparator_set_compare_value(pwm.comparator1, duty);
mcpwm_generator_set_action_on_timer_event(pwm.generator1,
MCPWM_GEN_TIMER_EVENT_ACTION(MCPWM_TIMER_DIRECTION_UP,
MCPWM_TIMER_EVENT_EMPTY, MCPWM_GEN_ACTION_HIGH));
mcpwm_generator_set_action_on_compare_event(pwm.generator1,
MCPWM_GEN_COMPARE_EVENT_ACTION(MCPWM_TIMER_DIRECTION_UP,
pwm.comparator1, MCPWM_GEN_ACTION_LOW));
mcpwm_timer_enable(pwm.timer);
mcpwm_timer_start_stop(pwm.timer, MCPWM_TIMER_START_NO_STOP);
return pwm;
}
void makeDCAC(int32_t gpio1, int32_t gpio2)
{
int32_t res = 1000000;
int32_t freq = 20000;
PWM pwm = makePWM(gpio1, res, freq, 10000);
pwm.generator2 = makeGen(pwm.oper, gpio2);
int deadtime = 200;
mcpwm_dead_time_config_t dtconfig = {
.posedge_delay_ticks = deadtime,
.negedge_delay_ticks = 0,
.flags.invert_output = false};
mcpwm_generator_set_dead_time(pwm.generator1,
pwm.generator1, &dtconfig);
dtconfig.posedge_delay_ticks = 0;
dtconfig.negedge_delay_ticks = deadtime;
dtconfig.flags.invert_output = true;
mcpwm_generator_set_dead_time(pwm.generator1, pwm.generator2,
&dtconfig);
}
void app_main(void)
{
makeDCAC(2,4);
}
Page 197-198 uses MCPWM.h given earlier
#include <stdio.h>
#include "freertos/FreeRTOS.h"
#include "driver/mcpwm_prelude.h"
#include "MCPWM.h"
void delay_ms(int t) {
vTaskDelay(t / portTICK_PERIOD_MS);
}
typedef struct {
mcpwm_timer_handle_t timer;
mcpwm_oper_handle_t oper;
mcpwm_gen_handle_t generator1;
mcpwm_gen_handle_t generator2;
mcpwm_cmpr_handle_t comparator1;
int32_t freq;
}PWM;
PWM makePWM(int gpio, uint32_t res, uint32_t ticksperiod,
uint32_t duty) {
PWM pwm;
pwm.timer = makeTimer(res, ticksperiod);
pwm.oper = makeOper();
mcpwm_operator_connect_timer(pwm.oper, pwm.timer);
pwm.generator1 = makeGen(pwm.oper, gpio);
pwm.comparator1 = makeComp(pwm.oper);
mcpwm_comparator_set_compare_value(pwm.comparator1, duty);
mcpwm_generator_set_action_on_timer_event(pwm.generator1,
MCPWM_GEN_TIMER_EVENT_ACTION(MCPWM_TIMER_DIRECTION_UP,
MCPWM_TIMER_EVENT_EMPTY, MCPWM_GEN_ACTION_HIGH));
mcpwm_generator_set_action_on_compare_event(pwm.generator1,
MCPWM_GEN_COMPARE_EVENT_ACTION(MCPWM_TIMER_DIRECTION_UP,
pwm.comparator1, MCPWM_GEN_ACTION_LOW));
mcpwm_timer_enable(pwm.timer);
mcpwm_timer_start_stop(pwm.timer, MCPWM_TIMER_START_NO_STOP);
return pwm;
}
PWM makeServo(int gpio) {
int32_t res = 1000000;
int32_t freq = 20000;
return makePWM(gpio, res, freq, 500);
}
void setAngle(PWM pwm, float angle) {
int32_t max = 2500;
int32_t min = 500;
int32_t duty = (float)(max - min) * angle + min;
mcpwm_comparator_set_compare_value(pwm.comparator1, duty);
}
void app_main(void)
{
PWM pwm = makeServo(2);
setAngle(pwm, 1.0);
delay_ms(100);
fflush(stdout);
setAngle(pwm, 0.5);
delay_ms(100);
setAngle(pwm, 0.0);
}
Page199 uses MCPWM.h given earlier and only partially listed on page
#include <stdio.h>
#include "freertos/FreeRTOS.h"
#include "driver/mcpwm_prelude.h"
#include "MCPWM.h"
void delay_ms(int t) {
vTaskDelay(t / portTICK_PERIOD_MS);
}
typedef struct {
mcpwm_timer_handle_t timer;
mcpwm_oper_handle_t oper;
mcpwm_gen_handle_t generator1;
mcpwm_gen_handle_t generator2;
mcpwm_cmpr_handle_t comparator1;
int32_t freq;
}PWM;
PWM makePWM(int gpio, uint32_t res, uint32_t ticksperiod,
uint32_t duty) {
PWM pwm;
pwm.timer = makeTimer(res, ticksperiod);
pwm.oper = makeOper();
mcpwm_operator_connect_timer(pwm.oper, pwm.timer);
pwm.generator1 = makeGen(pwm.oper, gpio);
pwm.comparator1 = makeComp(pwm.oper);
mcpwm_comparator_set_compare_value(pwm.comparator1, duty);
mcpwm_generator_set_action_on_timer_event(pwm.generator1,
MCPWM_GEN_TIMER_EVENT_ACTION(MCPWM_TIMER_DIRECTION_UP,
MCPWM_TIMER_EVENT_EMPTY, MCPWM_GEN_ACTION_HIGH));
mcpwm_generator_set_action_on_compare_event(pwm.generator1,
MCPWM_GEN_COMPARE_EVENT_ACTION(MCPWM_TIMER_DIRECTION_UP,
pwm.comparator1, MCPWM_GEN_ACTION_LOW));
mcpwm_timer_enable(pwm.timer);
mcpwm_timer_start_stop(pwm.timer, MCPWM_TIMER_START_NO_STOP);
return pwm;
}
PWM makeServo(int gpio) {
int32_t res = 1000000;
int32_t freq = 20000;
return makePWM(gpio, res, freq, 500);
}
void setAngle(PWM pwm, float angle) {
int32_t max = 2500;
int32_t min = 500;
int32_t duty = (float)(max - min) * angle + min;
mcpwm_comparator_set_compare_value(pwm.comparator1, duty);
}
void app_main(void)
{
PWM pwm = makeServo(2);
setAngle(pwm, 1.0);
delay_ms(100);
fflush(stdout);
setAngle(pwm, 0.5);
delay_ms(100);
setAngle(pwm, 0.0);
}
Page 208
#include <stdio.h>
#include "freertos/FreeRTOS.h"
#include "driver/gpio.h"
#include "soc/gpio_reg.h"
#include <unistd.h>
void gpio_set(int32_t value, int32_t mask) {
int32_t* OutAdd = (int32_t*)GPIO_OUT_REG;
*OutAdd = (*OutAdd & ~mask) | (value & mask);;
}
void delay_us(int t) {
usleep(t);
}
typedef struct
{
int gpio;
int speed;
bool forward;
uint32_t gpiomask;
int phase;
} StepperBi;
uint32_t stepTable[8] = (uint32_t[8]){ 0x8, 0xC, 0x4, 0x6, 0x2, 0x3, 0x1, 0x9 };
/*
{1, 0, 0, 0},
{1, 1, 0, 0},
{0, 1, 0, 0},
{0, 1, 1, 0},
{0, 0, 1, 0},
{0, 0, 1, 1},
{0, 0, 0, 1},
{1, 0, 0, 1}
*/
void StepperBiInit(StepperBi* s, int gpio)
{
s->gpio = gpio;
for (int i = 0; i < 4; i++) {
gpio_reset_pin((s->gpio) + i);
gpio_set_direction((s->gpio) + i, GPIO_MODE_OUTPUT);
}
s->gpiomask = 0x0F << gpio;
volatile uint32_t mask = stepTable[0] << gpio;
gpio_set(mask, s->gpiomask);
s->phase = 0;
s->speed = 0;
s->forward = true;
}
void setPhase(StepperBi* s, int p)
{
uint32_t mask = stepTable[p] << (s->gpio);
gpio_set(mask, s->gpiomask);
}
void stepForward(StepperBi* s)
{
s->phase = (s->phase + 1) % 8;
setPhase(s, s->phase);
}
void stepReverse(StepperBi* s)
{
s->phase = (s->phase - 1) % 8;
setPhase(s, s->phase);
}
void rotate(StepperBi* s, bool dir, int speed)
{
s->forward = dir;
s->speed = speed;
}
void app_main(void)
{
StepperBi s1;
StepperBiInit(&s1, 15);
while (true)
{
stepForward(&s1);
delay_us(1000);
}
}
Page 213
#include <stdio.h>
#include "freertos/FreeRTOS.h"
#include "driver/gpio.h"
#include "soc/gpio_reg.h"
#include <unistd.h>
#include "driver/gptimer.h"
void gpio_set(int32_t value, int32_t mask) {
int32_t* OutAdd = (int32_t*)GPIO_OUT_REG;
*OutAdd = (*OutAdd & ~mask) | (value & mask);;
}
void delay_ms(int t) {
vTaskDelay(t / portTICK_PERIOD_MS);
}
void delay_us(int t) {
usleep(t);
}
typedef struct
{
int gpio;
int speed;
bool forward;
uint32_t gpiomask;
int phase;
} StepperBi;
uint32_t stepTable[8] = (uint32_t[8]){ 0x8, 0xC, 0x4, 0x6, 0x2, 0x3, 0x1, 0x9 };
/*
{1, 0, 0, 0},
{1, 1, 0, 0},
{0, 1, 0, 0},
{0, 1, 1, 0},
{0, 0, 1, 0},
{0, 0, 1, 1},
{0, 0, 0, 1},
{1, 0, 0, 1}
*/
void StepperBiInit(StepperBi* s, int gpio)
{
s->gpio = gpio;
for (int i = 0; i < 4; i++) {
gpio_reset_pin((s->gpio) + i);
gpio_set_direction((s->gpio) + i, GPIO_MODE_OUTPUT);
}
s->gpiomask = 0x0F << gpio;
volatile uint32_t mask = stepTable[0] << gpio;
gpio_set(mask, s->gpiomask);
s->phase = 0;
s->speed = 0;
s->forward = true;
}
void setPhase(StepperBi* s, int p)
{
uint32_t mask = stepTable[p] << (s->gpio);
gpio_set(mask, s->gpiomask);
}
void stepForward(StepperBi* s)
{
s->phase = (s->phase + 1) % 8;
setPhase(s, s->phase);
}
void stepReverse(StepperBi* s)
{
s->phase = (s->phase - 1) % 8;
setPhase(s, s->phase);
}
bool step(gptimer_handle_t gptimer, const gptimer_alarm_event_data_t* edata, void* user_data)
{
StepperBi* s = (StepperBi*)(user_data);
if (s->forward)
{
stepForward(s);
}
else
{
stepReverse(s);
}
return false;
}
void rotate(StepperBi* s, bool dir, int speed)
{
static gptimer_handle_t gptimer = NULL;
if (gptimer == NULL) {
gptimer_config_t timer_config = {
.clk_src = GPTIMER_CLK_SRC_DEFAULT,
.direction = GPTIMER_COUNT_UP,
.resolution_hz = 1000000,
};
gptimer_new_timer(&timer_config, &gptimer);
gptimer_alarm_config_t alarm_config = {
.flags.auto_reload_on_alarm = true,
.alarm_count = 1000,
.reload_count = 0 };
gptimer_set_alarm_action(gptimer, &alarm_config);
gptimer_event_callbacks_t cbs = {
.on_alarm = step,
};
gptimer_register_event_callbacks(gptimer, &cbs, (void*)s);
gptimer_enable(gptimer);
gptimer_start(gptimer);
};
s->forward = dir;
if (speed == 0)
{
gptimer_stop(gptimer);
gptimer_disable(gptimer);
gptimer_del_timer(gptimer);
gptimer = NULL;
s->speed = 0;
return;
}
gptimer_alarm_config_t alarm_config = {
.flags.auto_reload_on_alarm = true,
.alarm_count = 2000,
.reload_count = 0 };
alarm_config.alarm_count = speed / 150.0 * 1000.0;
s->speed = speed;
gptimer_set_alarm_action(gptimer, &alarm_config);
}
void app_main(void)
{
StepperBi s1;
StepperBiInit(&s1, 15);
while (true)
{
rotate(&s1, true, 150);
delay_ms(100);
rotate(&s1, true, 00);
delay_ms(100);
}
}
Page 229
#include <stdio.h>
#include "freertos/FreeRTOS.h"
#include "driver/spi_common.h"
#include "driver/spi_master.h"
void app_main(void)
{
spi_bus_config_t busConfig = {
.sclk_io_num = 14,
.mosi_io_num = 13,
.miso_io_num = 12,
.quadwp_io_num = -1,
.quadhd_io_num = -1,
};
spi_bus_initialize(SPI2_HOST, &busConfig, SPI_DMA_DISABLED);
spi_device_interface_config_t masterConfig = {
.command_bits = 0,
.address_bits = 0,
.dummy_bits = 0,
.mode = 0,
.queue_size = 10,
.clock_speed_hz = SPI_MASTER_FREQ_8M,
.spics_io_num = 15,
};
spi_device_handle_t SPI = NULL;
spi_bus_add_device(SPI2_HOST, &masterConfig, &SPI);
spi_transaction_t transConfig = {
.length = 8 * 4,
.flags = SPI_TRANS_USE_RXDATA | SPI_TRANS_USE_TXDATA,
.tx_data = {0xAA, 0xAA, 0xAA, 0xAA},
};
spi_device_polling_transmit(SPI, &transConfig);
printf("received %X %X %X %X\n", transConfig.rx_data[0],
transConfig.rx_data[1], transConfig.rx_data[2],
transConfig.rx_data[3]);
}
Page 235
#include <stdio.h>
#include "freertos/FreeRTOS.h"
#include "driver/spi_common.h"
#include "driver/spi_master.h"
void app_main(void)
{
spi_bus_config_t busConfig = {
.sclk_io_num = 14,
.mosi_io_num = 13,
.miso_io_num = 12,
.quadwp_io_num = -1,
.quadhd_io_num = -1,
};
spi_bus_initialize(SPI2_HOST, &busConfig, SPI_DMA_DISABLED);
spi_device_interface_config_t masterConfig = {
.command_bits = 0,
.address_bits = 0,
.dummy_bits = 0,
.mode = 0,
.queue_size = 10,
.clock_speed_hz = 500000,
.spics_io_num = 15,
};
spi_device_handle_t SPI = NULL;
spi_bus_add_device(SPI2_HOST, &masterConfig, &SPI);
spi_transaction_t transConfig = {
.flags = SPI_TRANS_USE_RXDATA | SPI_TRANS_USE_TXDATA,
};
transConfig.tx_data[0] = 0x01;
transConfig.tx_data[1] = 0x80;
transConfig.tx_data[2] = 0x00;
transConfig.length = 8 * 3;
while (true)
{
spi_device_polling_transmit(SPI, &transConfig);
printf("received %X %X %X %X\n", transConfig.rx_data[0],
transConfig.rx_data[1], transConfig.rx_data[2],
transConfig.rx_data[3]);
int data = (transConfig.rx_data[1] & 0x03) << 8 |
transConfig.rx_data[2];
float volts = data * 3.3 / 1023.0;
printf("%f\n", volts);
fflush(stdout);
vTaskDelay(1000 / portTICK_PERIOD_MS);
}
}
Page 236-237 from fragments
#include <stdio.h>
#include "freertos/FreeRTOS.h"
#include "driver/spi_common.h"
#include "driver/spi_master.h"
spi_device_handle_t SPI_init()
{
spi_bus_config_t busConfig = {
.sclk_io_num = 14,
.mosi_io_num = 13,
.miso_io_num = 12,
.quadwp_io_num = -1,
.quadhd_io_num = -1,
};
spi_bus_initialize(SPI2_HOST, &busConfig, SPI_DMA_DISABLED);
spi_device_interface_config_t masterConfig = {
.command_bits = 0,
.address_bits = 0,
.dummy_bits = 0,
.mode = 0,
.queue_size = 10,
.clock_speed_hz = 500000,
.spics_io_num = 15,
};
spi_device_handle_t SPI = NULL;
spi_bus_add_device(SPI2_HOST, &masterConfig, &SPI);
return SPI;
}
float readADC(spi_device_handle_t SPI, uint8_t chan)
{
spi_transaction_t transConfig = {
.flags = SPI_TRANS_USE_RXDATA | SPI_TRANS_USE_TXDATA,
};
transConfig.tx_data[0] = 0x01;
transConfig.tx_data[1] = (0x08 | chan) << 4;
transConfig.tx_data[2] = 0x00;
transConfig.length = 8 * 3;
spi_device_polling_transmit(SPI, &transConfig);
int data = (transConfig.rx_data[1] & 0x03) << 8 |
transConfig.rx_data[2];
float volts = data * 3.3 / 1023.0;
return volts;
}
void app_main(void)
{
spi_device_handle_t SPI = SPI_init();
while (true) {
float volts = readADC(SPI, 0);
printf("%f\n", volts);
fflush(stdout);
vTaskDelay(1000 / portTICK_PERIOD_MS);
}
}
Page 247
#include <stdio.h>
#include "freertos/FreeRTOS.h"
#include "hal/adc_types.h"
#include "esp_adc/adc_oneshot.h"
void app_main(void)
{
adc_oneshot_unit_handle_t adc1_handle;
adc_oneshot_unit_init_cfg_t init_config1 = {
.unit_id = ADC_UNIT_1,
.ulp_mode = ADC_ULP_MODE_DISABLE,
};
adc_oneshot_new_unit(&init_config1, &adc1_handle);
adc_oneshot_chan_cfg_t config = {
.bitwidth = ADC_BITWIDTH_DEFAULT,
};
adc_oneshot_config_channel(adc1_handle, ADC_CHANNEL_6, &config);
int data;
while (true)
{
adc_oneshot_read(adc1_handle, ADC_CHANNEL_6, &data);
printf("%d\n", data);
fflush(stdout);
vTaskDelay(1000 / portTICK_PERIOD_MS);
}
}
Page 248
#include <stdio.h>
#include "freertos/FreeRTOS.h"
#include "hal/adc_types.h"
#include "esp_adc/adc_oneshot.h"
#include "esp_adc/adc_cali.h"
#include "esp_adc/adc_cali_scheme.h"
void app_main(void)
{
adc_cali_scheme_ver_t scheme_mask;
adc_cali_check_scheme(&scheme_mask);
if (scheme_mask & ADC_CALI_SCHEME_VER_CURVE_FITTING)
{
printf("Curve Fit %d\n", scheme_mask);
}
else
{
if (scheme_mask & ADC_CALI_SCHEME_VER_LINE_FITTING)
{
printf("Line Fit %d\n", scheme_mask);
}
}
}
Page 249
#include <stdio.h>
#include "freertos/FreeRTOS.h"
#include "hal/adc_types.h"
#include "esp_adc/adc_oneshot.h"
#include "esp_adc/adc_cali.h"
#include "esp_adc/adc_cali_scheme.h"
void app_main(void)
{
adc_oneshot_unit_handle_t adc1_handle;
adc_oneshot_unit_init_cfg_t init_config1 = {
.unit_id = ADC_UNIT_1,
.ulp_mode = ADC_ULP_MODE_DISABLE,
};
adc_oneshot_new_unit(&init_config1, &adc1_handle);
adc_oneshot_chan_cfg_t config = {
.bitwidth = ADC_BITWIDTH_12,
.atten = ADC_ATTEN_DB_0};
adc_oneshot_config_channel(adc1_handle, ADC_CHANNEL_6, &config);
/* adc_cali_line_fitting_config_t calfit = {
.unit_id = ADC_UNIT_1,
.atten = ADC_ATTEN_DB_0,
.bitwidth = ADC_BITWIDTH_12
}; */
adc_cali_curve_fitting_config_t calfit = {
.unit_id = ADC_UNIT_1,
.atten = ADC_ATTEN_DB_0,
.bitwidth = ADC_BITWIDTH_12};
adc_cali_handle_t cali_handle;
// adc_cali_create_scheme_line_fitting(&calfit, &cali_handle);
adc_cali_create_scheme_curve_fitting(&calfit, &cali_handle);
int data;
while (true)
{
adc_oneshot_get_calibrated_result(adc1_handle, cali_handle,
ADC_CHANNEL_6, &data);
printf("data cal %d mV\n", data);
fflush(stdout);
vTaskDelay(1000 / portTICK_PERIOD_MS);
}
}
Page 251 From fragement
#include <stdio.h>
#include "freertos/FreeRTOS.h"
#include "hal/adc_types.h"
#include "esp_adc/adc_oneshot.h"
#include "esp_adc/adc_cali.h"
#include "esp_adc/adc_cali_scheme.h"
#include "driver/gptimer.h"
gptimer_handle_t tick_us_start(void)
{
gptimer_config_t timer_config = {
.clk_src = GPTIMER_CLK_SRC_DEFAULT,
.direction = GPTIMER_COUNT_UP,
.resolution_hz = 1000000,
};
gptimer_handle_t gptimer_us = NULL;
gptimer_new_timer(&timer_config, &gptimer_us);
gptimer_enable(gptimer_us);
gptimer_start(gptimer_us);
return gptimer_us;
}
int64_t tick_us(gptimer_handle_t gptimer_us, int64_t offset)
{
uint64_t count;
gptimer_get_raw_count(gptimer_us, &count);
return count + offset;
}
void app_main(void)
{
adc_oneshot_unit_handle_t adc1_handle;
adc_oneshot_unit_init_cfg_t init_config1 = {
.unit_id = ADC_UNIT_1,
.ulp_mode = ADC_ULP_MODE_DISABLE,
};
adc_oneshot_new_unit(&init_config1, &adc1_handle);
adc_oneshot_chan_cfg_t config = {
.bitwidth = ADC_BITWIDTH_12,
.atten = ADC_ATTEN_DB_0};
adc_oneshot_config_channel(adc1_handle, ADC_CHANNEL_6, &config);
/* adc_cali_line_fitting_config_t calfit = {
.unit_id = ADC_UNIT_1,
.atten = ADC_ATTEN_DB_0,
.bitwidth = ADC_BITWIDTH_12
}; */
adc_cali_curve_fitting_config_t calfit = {
.unit_id = ADC_UNIT_1,
.atten = ADC_ATTEN_DB_0,
.bitwidth = ADC_BITWIDTH_12};
adc_cali_handle_t cali_handle;
// adc_cali_create_scheme_line_fitting(&calfit, &cali_handle);
adc_cali_create_scheme_curve_fitting(&calfit, &cali_handle);
gptimer_handle_t timer = tick_us_start();
int data;
int64_t t = tick_us(timer, 0);
for (int i = 0; i < 100000; i++)
{
adc_oneshot_read(adc1_handle, ADC_CHANNEL_6, &data);
}
t = tick_us(timer, 0) - t;
printf("t=%lld \n", t / 100000);
}
Page 252 Note: Only works on an ESP32 or ESP32 S2
#include <stdio.h>
#include "freertos/FreeRTOS.h"
#include "driver/dac_oneshot.h"
void app_main(void)
{
dac_oneshot_config_t oneshot_cfg = {
.chan_id = DAC_CHAN_1
};
dac_oneshot_handle_t DAC_handle;
dac_oneshot_new_channel(&oneshot_cfg, &DAC_handle);
uint8_t value = 0;
while (true) {
dac_oneshot_output_voltage(DAC_handle, value);
value++;
}
}
Page 254 Note: Only works on an ESP32 or ESP32 S2
#include <stdio.h>
#include "freertos/FreeRTOS.h"
#include "driver/dac_oneshot.h"
#include "math.h"
uint8_t wave[256];
void app_main(void)
{
for (int i = 0; i < 256; i++)
{
wave[i] = (uint8_t)((128.0 + 127 * sinf((float)i
* 2.0 * 3.14159 / 255.0)));
}
dac_oneshot_config_t oneshot_cfg = {
.chan_id = DAC_CHAN_1
};
dac_oneshot_handle_t DAC_handle;
dac_oneshot_new_channel(&oneshot_cfg, &DAC_handle);
while (true) {
for (int i = 0;i < 256;i++) {
dac_oneshot_output_voltage(DAC_handle, wave[i]);
}
}
}
Page 255 Note: Only works on an ESP32 or ESP32 S2
#include <stdio.h>
#include "freertos/FreeRTOS.h"
#include "driver/dac_oneshot.h"
#include "math.h"
uint8_t wave[256];
void app_main(void)
{
for (int i = 0; i < 256; i++)
{
wave[i] = (uint8_t)((128.0 + 127 * sinf((float)i
* 2.0 * 3.14159 / 255.0)));
}
dac_oneshot_config_t oneshot_cfg = {
.chan_id = DAC_CHAN_1
};
dac_oneshot_handle_t DAC_handle;
dac_oneshot_new_channel(&oneshot_cfg, &DAC_handle);
while (true) {
for (int i = 0;i < 256;i++) {
dac_oneshot_output_voltage(DAC_handle, wave[i]);
}
}
}
Page 259 ESP32 only
#include <stdio.h>
#include "freertos/FreeRTOS.h"
#include "driver/touch_sensor.h"
void app_main(void)
{
touch_pad_init();
touch_pad_set_voltage(TOUCH_HVOLT_2V4,
TOUCH_LVOLT_0V5, TOUCH_HVOLT_ATTEN_1V);
touch_pad_config(TOUCH_PAD_NUM5, 0);
uint16_t touch_value=0;
while (true) {
touch_pad_read(TOUCH_PAD_NUM5, &touch_value);
printf("val_touch_gpio12 = %d \n", touch_value);
vTaskDelay(1000 / portTICK_PERIOD_MS);
}
}
Page 259 ESP32 S3 only
#include <stdio.h>
#include "freertos/FreeRTOS.h"
#include "driver/touch_sensor.h"
void app_main(void)
{
touch_pad_init();
touch_pad_config(TOUCH_PAD_NUM5);
touch_pad_set_fsm_mode(TOUCH_FSM_MODE_SW);
uint32_t touch_value = 0;
while (true)
{
touch_pad_sw_start();
vTaskDelay(1000 / portTICK_PERIOD_MS);
touch_pad_read_raw_data(TOUCH_PAD_NUM5, &touch_value);
printf("val_touch_gpio5 = %ld \n", touch_value);
}
}
Page 260 ESP32 only
#include <stdio.h>
#include "freertos/FreeRTOS.h"
#include "driver/touch_sensor.h"
#include "driver/gpio.h"
void ISR(void *arg)
{
gpio_set_level(2, !gpio_get_level(2));
}
void app_main(void)
{
gpio_reset_pin(2);
gpio_set_direction(2, GPIO_MODE_INPUT_OUTPUT);
touch_pad_init();
touch_pad_set_voltage(TOUCH_HVOLT_2V4, TOUCH_LVOLT_0V5,
TOUCH_HVOLT_ATTEN_1V);
touch_pad_config(TOUCH_PAD_NUM5, 800);
touch_pad_set_trigger_mode(TOUCH_TRIGGER_BELOW);
touch_pad_isr_register(ISR, NULL);
touch_pad_intr_enable();
uint16_t touch_value = 0;
while (true)
{
uint32_t status = touch_pad_get_status();
touch_pad_read(TOUCH_PAD_NUM5, &touch_value);
printf("val_touch_gpio12 = %d \n", touch_value);
printf("status = %ld\n", status);
vTaskDelay(1000 / portTICK_PERIOD_MS);
}
}
Page 262 ESP32 S3 only
#include <stdio.h>
#include "freertos/FreeRTOS.h"
#include "driver/touch_sensor.h"
#include "driver/gpio.h"
void ISR(void *arg)
{
gpio_set_level(2, !gpio_get_level(2));
touch_pad_clear_status();
}
void app_main(void)
{
gpio_reset_pin(2);
gpio_set_direction(2, GPIO_MODE_INPUT_OUTPUT);
touch_pad_init();
touch_pad_config(TOUCH_PAD_NUM5);
touch_pad_set_thresh(TOUCH_PAD_NUM5, 30000);
touch_pad_set_channel_mask(1 << 5);
touch_pad_isr_register(ISR, NULL, TOUCH_PAD_INTR_MASK_ALL);
touch_pad_intr_enable(TOUCH_PAD_INTR_MASK_ACTIVE);
touch_pad_set_fsm_mode(TOUCH_FSM_MODE_TIMER);
Page 274
#include <stdio.h>
#include "freertos/FreeRTOS.h"
#include "driver/i2c_master.h"
void app_main(void)
{
i2c_master_bus_config_t i2cBus = {
.i2c_port = I2C_NUM_0,
.scl_io_num = 15,
.sda_io_num = 16,
.clk_source = I2C_CLK_SRC_DEFAULT,
.glitch_ignore_cnt = 7,
.flags.enable_internal_pullup = true};
i2c_master_bus_handle_t bus_handle;
i2c_new_master_bus(&i2cBus, &bus_handle);
i2c_device_config_t i2cdev = {
.dev_addr_length = I2C_ADDR_BIT_LEN_7,
.device_address = 0x40,
.scl_speed_hz = 100000,
.scl_wait_us = 10};
i2c_master_dev_handle_t dev_handle;
i2c_master_bus_add_device(bus_handle, &i2cdev, &dev_handle);
uint8_t buf[10] = {0xE7};
i2c_master_transmit(dev_handle, buf, 1, 1000);
i2c_master_receive(dev_handle, buf, 1, 100);
printf("User Register = %X \r\n", buf[0]);
}
Page 278
#include <stdio.h>
#include "freertos/FreeRTOS.h"
#include "driver/i2c_master.h"
void app_main(void)
{
i2c_master_bus_config_t i2cBus = {
.i2c_port = I2C_NUM_0,
.scl_io_num = 15,
.sda_io_num = 16,
.clk_source = I2C_CLK_SRC_DEFAULT,
.glitch_ignore_cnt = 7,
.flags.enable_internal_pullup = true};
i2c_master_bus_handle_t bus_handle;
i2c_new_master_bus(&i2cBus, &bus_handle);
i2c_device_config_t i2cdev = {
.dev_addr_length = I2C_ADDR_BIT_LEN_7,
.device_address = 0x40,
.scl_speed_hz = 100000,
.scl_wait_us = 10};
i2c_master_dev_handle_t dev_handle;
i2c_master_bus_add_device(bus_handle, &i2cdev, &dev_handle);
uint8_t buf[10] = {0xF3};
i2c_master_transmit(dev_handle, buf, 1, 100);
esp_err_t err;
do
{
err = i2c_master_receive(dev_handle, buf, 3, 100);
if (err != ESP_OK)
{
i2c_master_bus_rm_device(dev_handle);
i2c_del_master_bus(bus_handle);
i2c_new_master_bus(&i2cBus, &bus_handle);
i2c_master_bus_add_device(bus_handle, &i2cdev, &dev_handle);
};
} while (err != ESP_OK);
uint8_t msb = buf[0];
uint8_t lsb = buf[1];
uint8_t check = buf[2];
printf("msb %d \n lsb %d \n checksum %d \n", msb, lsb, check);
fflush(stdout);
}
Page 278 complete program from fragment
#include <stdio.h>
#include "freertos/FreeRTOS.h"
#include "driver/i2c_master.h"
void app_main(void)
{
i2c_master_bus_config_t i2cBus = {
.i2c_port = I2C_NUM_0,
.scl_io_num = 15,
.sda_io_num = 16,
.clk_source = I2C_CLK_SRC_DEFAULT,
.glitch_ignore_cnt = 7,
.flags.enable_internal_pullup = true};
i2c_master_bus_handle_t bus_handle;
i2c_new_master_bus(&i2cBus, &bus_handle);
i2c_device_config_t i2cdev = {
.dev_addr_length = I2C_ADDR_BIT_LEN_7,
.device_address = 0x40,
.scl_speed_hz = 100000,
.scl_wait_us = 10};
i2c_master_dev_handle_t dev_handle;
i2c_master_bus_add_device(bus_handle, &i2cdev, &dev_handle);
uint8_t buf[10] = {0xF3};
i2c_master_transmit(dev_handle, buf, 1, 100);
esp_err_t err;
do
{
err = i2c_master_receive(dev_handle, buf, 3, 100);
vTaskDelay(10 / portTICK_PERIOD_MS);
} while (err != ESP_OK);
uint8_t msb = buf[0];
uint8_t lsb = buf[1];
uint8_t check = buf[2];
printf("msb %d \n lsb %d \n checksum %d \n", msb, lsb, check);
fflush(stdout);
}
Page 279 Complete program from fragment
#include <stdio.h>
#include "freertos/FreeRTOS.h"
#include "driver/i2c_master.h"
void app_main(void)
{
i2c_master_bus_config_t i2cBus = {
.i2c_port = I2C_NUM_0,
.scl_io_num = 15,
.sda_io_num = 16,
.clk_source = I2C_CLK_SRC_DEFAULT,
.glitch_ignore_cnt = 7,
.flags.enable_internal_pullup = true};
i2c_master_bus_handle_t bus_handle;
i2c_new_master_bus(&i2cBus, &bus_handle);
i2c_device_config_t i2cdev = {
.dev_addr_length = I2C_ADDR_BIT_LEN_7,
.device_address = 0x40,
.scl_speed_hz = 100000,
.scl_wait_us = 10};
i2c_master_dev_handle_t dev_handle;
i2c_master_bus_add_device(bus_handle, &i2cdev, &dev_handle);
uint8_t buf[10] = {0xF3};
i2c_master_transmit(dev_handle, buf, 1, 100);
vTaskDelay(50 / portTICK_PERIOD_MS);
i2c_master_receive(dev_handle, buf, 3, 100);
uint8_t msb = buf[0];
uint8_t lsb = buf[1];
uint8_t check = buf[2];
printf("msb %d \n lsb %d \n checksum %d \n", msb, lsb, check);
fflush(stdout);
}
Page 282
#include <stdio.h>
#include "freertos/FreeRTOS.h"
#include "driver/i2c_master.h"
uint8_t crcCheck(uint8_t msb, uint8_t lsb, uint8_t check)
{
uint32_t data32 = ((uint32_t)msb << 16) | ((uint32_t)lsb << 8) |
(uint32_t)check;
uint32_t divisor = 0x988000;
for (int i = 0; i < 16; i++)
{
if (data32 & (uint32_t)1 << (23 - i))
data32 ^= divisor;
divisor >>= 1;
};
return (uint8_t)data32;
}
void app_main(void)
{
i2c_master_bus_config_t i2cBus = {
.i2c_port = I2C_NUM_0,
.scl_io_num = 15,
.sda_io_num = 16,
.clk_source = I2C_CLK_SRC_DEFAULT,
.glitch_ignore_cnt = 7,
.flags.enable_internal_pullup = true};
i2c_master_bus_handle_t bus_handle;
i2c_new_master_bus(&i2cBus, &bus_handle);
i2c_device_config_t i2cdev = {
.dev_addr_length = I2C_ADDR_BIT_LEN_7,
.device_address = 0x40,
.scl_speed_hz = 100000,
.scl_wait_us = 10};
i2c_master_dev_handle_t dev_handle;
i2c_master_bus_add_device(bus_handle, &i2cdev, &dev_handle);
uint8_t buf[10] = {0xF3};
i2c_master_transmit(dev_handle, buf, 1, 100);
vTaskDelay(50 / portTICK_PERIOD_MS);
i2c_master_receive(dev_handle, buf, 3, 100);
uint8_t msb = buf[0];
uint8_t lsb = buf[1];
uint8_t check = buf[2];
unsigned int data16 = ((unsigned int)msb << 8) |
(unsigned int)(lsb & 0xFC);
float temp = (float)(-46.85 + (175.72 * data16 / (float)65536));
printf("Temperature %f C \n\r", temp);
printf("crc = %d\n\r", crcCheck(msb, lsb, check));
buf[0] = 0xF5;
i2c_master_transmit(dev_handle, buf, 1, 100);
vTaskDelay(50 / portTICK_PERIOD_MS);
i2c_master_receive(dev_handle, buf, 3, 100);
msb = buf[0];
lsb = buf[1];
check = buf[2];
data16 = ((unsigned int)msb << 8) | (unsigned int)(lsb & 0xFC);
float hum = -6 + (125.0 * (float)data16) / 65536;
printf("Humidity %f %% \n\r", hum);
printf("crc = %d\n\r", crcCheck(msb, lsb, check));
}
Page 292
#include <stdio.h>
#include "freertos/FreeRTOS.h"
#include "driver/gpio.h"
#include "driver/gptimer.h"
#include <unistd.h>
void delay_us(int t)
{
usleep(t);
}
gptimer_handle_t tick_us_start(void) {
gptimer_config_t timer_config = {
.clk_src = GPTIMER_CLK_SRC_DEFAULT,
.direction = GPTIMER_COUNT_UP,
.resolution_hz = 1000000,
};
gptimer_handle_t gptimer_us = NULL;
gptimer_new_timer(&timer_config, &gptimer_us);
gptimer_enable(gptimer_us);
gptimer_start(gptimer_us);
return gptimer_us;
}
int64_t tick_us(gptimer_handle_t gptimer_us, int64_t offset) {
uint64_t count;
gptimer_get_raw_count(gptimer_us, &count);
return count + offset;
}
void app_main(void)
{
gptimer_handle_t timer = tick_us_start();
gpio_reset_pin(2);
gpio_set_direction(2, GPIO_MODE_OUTPUT);
gpio_set_level(2, 1);
delay_us(1000);
gpio_set_level(2, 0);
delay_us(1000);
gpio_set_direction(2, GPIO_MODE_INPUT);
while (gpio_get_level(2) == 1)
{
};
while (gpio_get_level(2) == 0)
{
};
while (gpio_get_level(2) == 1)
{
};
int64_t t2;
uint32_t data = 0;
int64_t t1 = tick_us(timer, 0);
for (int i = 0; i < 32; i++)
{
while (gpio_get_level(2) == 0)
{
};
while (gpio_get_level(2) == 1)
{
};
t2 = tick_us(timer, 0);
data = data << 1;
data = data | ((t2 - t1) > 100);
t1 = t2;
}
uint8_t checksum = 0;
for (int i = 0; i < 8; i++)
{
while (gpio_get_level(2) == 0)
{
};
while (gpio_get_level(2) == 1)
{
};
t2 = tick_us(timer, 0);
checksum = checksum << 1;
checksum = checksum | ((t2 - t1) > 100);
t1 = t2;
}
printf("data %ld\n", data);
uint8_t byte1 = (data >> 24 & 0xFF);
uint8_t byte2 = (data >> 16 & 0xFF);
uint8_t byte3 = (data >> 8 & 0xFF);
uint8_t byte4 = (data & 0xFF);
printf("Checksum %X %X\n", checksum,
(byte1 + byte2 + byte3 + byte4) & 0xFF);
float humidity = (float)((byte1 << 8) | byte2) / 10.0;
printf("Humidity= %f %%\n", humidity);
float temperature;
int neg = byte3 & 0x80;
byte3 = byte3 & 0x7F;
temperature = (float)(byte3 << 8 | byte4) / 10.0;
if (neg > 0)
temperature = -temperature;
printf("Temperature= %f C\n", temperature);
}
Page 294 from fragment
#include <stdio.h>
#include "freertos/FreeRTOS.h"
#include "driver/gpio.h"
#include <unistd.h>
void delay_us(int t)
{
usleep(t);
}
void app_main(void)
{
gpio_reset_pin(2);
gpio_set_direction(2, GPIO_MODE_OUTPUT);
gpio_set_level(2, 1);
delay_us(1000);
gpio_set_level(2, 0);
delay_us(1000);
gpio_set_direction(2, GPIO_MODE_INPUT);
while (gpio_get_level(2) == 1)
{
};
while (gpio_get_level(2) == 0)
{
};
while (gpio_get_level(2) == 1)
{
};
uint32_t data = 0;
for (int i = 0; i < 32; i++)
{
while (gpio_get_level(2) == 0)
{
};
delay_us(50);
data = data << 1;
data = data | gpio_get_level(2);
while (gpio_get_level(2) == 1)
{
};
}
uint8_t checksum = 0;
for (int i = 0; i < 8; i++)
{
while (gpio_get_level(2) == 0)
{
};
delay_us(50);
checksum = checksum << 1;
checksum = checksum | gpio_get_level(2);
while (gpio_get_level(2) == 1)
{
};
}
printf("data %ld\n", data);
uint8_t byte1 = (data >> 24 & 0xFF);
uint8_t byte2 = (data >> 16 & 0xFF);
uint8_t byte3 = (data >> 8 & 0xFF);
uint8_t byte4 = (data & 0xFF);
printf("Checksum %X %X\n", checksum,
(byte1 + byte2 + byte3 + byte4) & 0xFF);
float humidity = (float)((byte1 << 8) | byte2) / 10.0;
printf("Humidity= %f %%\n", humidity);
float temperature;
int neg = byte3 & 0x80;
byte3 = byte3 & 0x7F;
temperature = (float)(byte3 << 8 | byte4) / 10.0;
if (neg > 0)
temperature = -temperature;
printf("Temperature= %f C\n", temperature);
}
Page 298
#include <stdio.h>
#include "freertos/FreeRTOS.h"
#include "driver/gpio.h"
#include "driver/rmt_rx.h"
#include <unistd.h>
TaskHandle_t taskhandle;
void delay_us(int t)
{
usleep(t);
}
bool rmtrxDone(rmt_channel_handle_t channel,
const rmt_rx_done_event_data_t *edata, void *user_data)
{
xTaskResumeFromISR(*(TaskHandle_t *)user_data);
return true;
}
rmt_symbol_word_t raw_symbols[100];
void app_main(void)
{
rmt_rx_channel_config_t rmtrxconfig = {
.gpio_num = 2,
.resolution_hz = 1000000,
.mem_block_symbols = 100,
.clk_src = RMT_CLK_SRC_DEFAULT,
.flags.invert_in = false,
.flags.with_dma = false,
};
rmt_channel_handle_t rmtrxHandle;
rmt_new_rx_channel(&rmtrxconfig, &rmtrxHandle);
rmt_rx_event_callbacks_t cbs = {
.on_recv_done = rmtrxDone};
taskhandle = xTaskGetCurrentTaskHandle();
rmt_rx_register_event_callbacks(rmtrxHandle, &cbs, &taskhandle);
rmt_enable(rmtrxHandle);
rmt_receive_config_t receive_config = {
.signal_range_min_ns = 2000,
.signal_range_max_ns = 900000,
};
while (true)
{
gpio_reset_pin(2);
gpio_set_direction(2, GPIO_MODE_OUTPUT);
gpio_set_level(2, 1);
delay_us(1000);
gpio_set_level(2, 0);
delay_us(1000);
gpio_set_direction(2, GPIO_MODE_INPUT);
while (gpio_get_level(2) == 1)
{
};
while (gpio_get_level(2) == 0)
{
};
while (gpio_get_level(2) == 1)
{
};
rmt_receive(rmtrxHandle, raw_symbols, sizeof(raw_symbols),
&receive_config);
vTaskSuspend(taskhandle);
uint8_t byte1 = 0;
uint8_t byte2 = 0;
uint8_t byte3 = 0;
uint8_t byte4 = 0;
uint8_t checksum = 0;
for (int i = 0; i < 40; i = i + 1)
{
switch (i / 8)
{
case 0:
byte1 = byte1 << 1;
byte1 = byte1 | (raw_symbols[i].duration0 > 50);
break;
case 1:
byte2 = byte2 << 1;
byte2 = byte2 | (raw_symbols[i].duration0 > 50);
break;
case 2:
byte3 = byte3 << 1;
byte3 = byte3 | (raw_symbols[i].duration0 > 50);
break;
case 3:
byte4 = byte4 << 1;
byte4 = byte4 | (raw_symbols[i].duration0 > 50);
break;
case 4:
checksum = checksum << 1;
checksum = checksum | (raw_symbols[i].duration0 > 50);
break;
}
}
printf("Checksum %X %X\n", checksum,
(byte1 + byte2 + byte3 + byte4) & 0xFF);
float humidity = (float)((byte1 << 8) | byte2) / 10.0;
printf("Humidity= %f %%\n", humidity);
float temperature;
int neg = byte3 & 0x80;
byte3 = byte3 & 0x7F;
temperature = (float)(byte3 << 8 | byte4) / 10.0;
if (neg > 0)
temperature = -temperature;
printf("Temperature= %f C\n", temperature);
delay_us(1000000);
}
}
Page 311
#include <stdio.h>
#include "freertos/FreeRTOS.h"
#include "driver/gpio.h"
#include <unistd.h>
void delay_us(int t)
{
usleep(t);
}
uint8_t readBit(uint8_t pin)
{
gpio_set_level(pin, 0);
delay_us(2);
gpio_set_level(pin, 1);
delay_us(5);
uint8_t b = gpio_get_level(pin);
delay_us(60);
return b;
}
void writeBit(uint8_t pin, int b)
{
int delay1, delay2;
if (b == 1)
{
delay1 = 6;
delay2 = 64;
}
else
{
delay1 = 60;
delay2 = 10;
}
gpio_set_level(pin, 0);
delay_us(delay1);
gpio_set_level(pin, 1);
delay_us(delay2);
}
int readByte(uint8_t pin)
{
int byte = 0;
for (int i = 0; i < 8; i++)
{
byte = byte | readBit(pin) << i;
};
return byte;
}
void writeByte(uint8_t pin, int byte)
{
for (int i = 0; i < 8; i++)
{
if (byte & 1)
{
writeBit(pin, 1);
}
else
{
writeBit(pin, 0);
}
byte = byte >> 1;
}
}
int presence(int pin)
{
gpio_reset_pin(pin);
gpio_set_direction(pin, GPIO_MODE_INPUT_OUTPUT_OD);
gpio_set_level(pin, 1);
delay_us(1000);
gpio_set_level(pin, 0);
delay_us(480);
gpio_set_level(pin, 1);
delay_us(70);
int res = gpio_get_level(pin);
delay_us(410);
return res;
}
int convert(uint8_t pin)
{
writeByte(pin, 0x44);
int i;
for (i = 0; i < 500; i++)
{
delay_us(10000);
if (readBit(pin) == 1)
break;
}
return i;
}
uint8_t crc8(uint8_t *data, uint8_t len)
{
uint8_t temp;
uint8_t databyte;
uint8_t crc = 0;
for (int i = 0; i < len; i++)
{
databyte = data[i];
for (int j = 0; j < 8; j++)
{
temp = (crc ^ databyte) & 0x01;
crc >>= 1;
if (temp)
crc ^= 0x8C;
databyte >>= 1;
}
}
return crc;
}
float getTemperature(uint8_t pin)
{
if (presence(pin) == 1)
return -1000;
writeByte(pin, 0xCC);
if (convert(pin) == 500)
return -3000;
presence(pin);
writeByte(pin, 0xCC);
writeByte(pin, 0xBE);
uint8_t data[9];
for (int i = 0; i < 9; i++)
{
data[i] = readByte(pin);
}
uint8_t crc = crc8(data, 9);
if (crc != 0)
return -2000;
int t1 = data[0];
int t2 = data[1];
int16_t temp1 = (t2 << 8 | t1);
float temp = (float)temp1 / 16;
return temp;
}
void app_main(void)
{
while (true)
{
float temp = getTemperature(2);
printf("temperature=%f\n", temp);
delay_us(1000000);
}
}
Page 318 ESP32 S3
#include "driver/gpio.h"
#include "freertos/FreeRTOS.h"
void writeGRB(int pin, int GRB)
{
int mask = 0x800000;
volatile int duty = 0;
for (int j = 0; j < 24; j++)
{
gpio_set_level(pin, 1);
if ((GRB & mask))
{
for (volatile int i = 0; i < 3; i++)
{
}
duty = 0;
duty = 0;
gpio_set_level(pin, 0);
duty++;
duty = 0;
duty = 0;
duty = 0;
}
else
{
duty = 0;
gpio_set_level(pin, 0);
for (volatile int i = 0; i < 5; i++)
{
}
}
mask = mask >> 1;
}
vTaskDelay(60 / portTICK_PERIOD_MS);
}
void app_main(void)
{
int pin = 48;
gpio_reset_pin(pin);
gpio_set_direction(pin, GPIO_MODE_OUTPUT);
gpio_set_level(pin, 0);
vTaskDelay(10 / portTICK_PERIOD_MS);
int color = 0x0000FF;
writeGRB(pin, color);
vTaskDelay(1000 / portTICK_PERIOD_MS);
color = 0x00FF00;
writeGRB(pin, color);
vTaskDelay(1000 / portTICK_PERIOD_MS);
color = 0xFF0000;
writeGRB(pin, color);
vTaskDelay(1000 / portTICK_PERIOD_MS);
}
Page 319 complete program
#include "driver/gpio.h"
#include "freertos/FreeRTOS.h"
void writeGRB(int pin, int GRB)
{
int mask = 0x800000;
volatile int duty = 0;
for (int j = 0; j < 24; j++)
{
gpio_set_level(pin, 1);
if ((GRB & mask))
{
for (volatile int i = 0; i < 2; i++)
{
}
duty = 0;
gpio_set_level(pin, 0);
duty = 0;
duty = 0;
duty = 0;
duty = 0;
}
else
{
gpio_set_level(pin, 0);
for (volatile int i = 0; i < 3; i++)
{
}
duty = 0;
}
vTaskDelay(60 / portTICK_PERIOD_MS);
}
}
void app_main(void)
{
int pin = 48;
gpio_reset_pin(pin);
gpio_set_direction(pin, GPIO_MODE_OUTPUT);
gpio_set_level(pin, 0);
vTaskDelay(10 / portTICK_PERIOD_MS);
int color = 0x0000FF;
writeGRB(pin, color);
vTaskDelay(1000 / portTICK_PERIOD_MS);
color = 0x00FF00;
writeGRB(pin, color);
vTaskDelay(1000 / portTICK_PERIOD_MS);
color = 0xFF0000;
writeGRB(pin, color);
vTaskDelay(1000 / portTICK_PERIOD_MS);
}
Page 321
#include "driver/gpio.h"
#include "freertos/FreeRTOS.h"
#include "driver/rmt_tx.h"
void convertGRB(int GRB, rmt_symbol_word_t *rawdata)
{
int mask = 0x800000;
for (int j = 0; j < 24; j++)
{
if ((GRB & mask))
{
rawdata[j].level0 = 1;
rawdata[j].duration0 = 7;
rawdata[j].level1 = 0;
rawdata[j].duration1 = 12 - 7;
}
else
{
rawdata[j].level0 = 1;
rawdata[j].duration0 = 3;
rawdata[j].level1 = 0;
rawdata[j].duration1 = 12 - 3;
}
mask = mask >> 1;
}
}
rmt_symbol_word_t raw_symbols[100];
void app_main(void)
{
int pin = 2;
rmt_channel_handle_t led_chan = NULL;
rmt_tx_channel_config_t tx_chan_config = {
.clk_src = RMT_CLK_SRC_DEFAULT,
.gpio_num = pin,
.mem_block_symbols = 64,
.resolution_hz = 10000000,
.trans_queue_depth = 4,
.flags.with_dma = false,
};
rmt_new_tx_channel(&tx_chan_config, &led_chan);
rmt_copy_encoder_config_t config = {};
rmt_encoder_handle_t encoder = NULL;
rmt_new_copy_encoder(&config, &encoder);
rmt_enable(led_chan);
rmt_transmit_config_t txconf = {
.loop_count = 1};
int color = 0x00FFFF;
convertGRB(color, raw_symbols);
rmt_transmit(led_chan, encoder, raw_symbols, 24 * sizeof(rmt_symbol_word_t), &txconf);
rmt_tx_wait_all_done(led_chan, 10000);
}
Page 330
#include <stdio.h>
#include "freertos/FreeRTOS.h"
#include <unistd.h>
#include "driver/uart.h"
void delay_us(int t)
{
usleep(t);
}
void app_main(void)
{
uart_driver_install(UART_NUM_2, 1024, 1024, 0, NULL, 0);
uart_config_t uart_config = {
.baud_rate = 9600,
.data_bits = UART_DATA_8_BITS,
.parity = UART_PARITY_DISABLE,
.stop_bits = UART_STOP_BITS_1,
.flow_ctrl = UART_HW_FLOWCTRL_DISABLE,
.source_clk = UART_SCLK_DEFAULT};
uart_param_config(UART_NUM_2, &uart_config);
uart_set_pin(UART_NUM_2, 1, 2, -1, -1);
char SendData[] = "Hello World";
uart_flush(UART_NUM_2);
uart_write_bytes(UART_NUM_2, SendData, sizeof(SendData));
char RecData[100];
int n = uart_read_bytes(UART_NUM_2, RecData,
sizeof(SendData), 3);
RecData[n + 1] = 0;
printf("%s\n", RecData);
printf("%d %d\n", n, sizeof(SendData));
}
Page 333
#include <stdio.h>
#include "freertos/FreeRTOS.h"
#include "driver/uart.h"
#include "driver/gptimer.h"
gptimer_handle_t tick_us_start(void)
{
gptimer_config_t timer_config = {
.clk_src = GPTIMER_CLK_SRC_DEFAULT,
.direction = GPTIMER_COUNT_UP,
.resolution_hz = 1000000,
};
gptimer_handle_t gptimer_us = NULL;
gptimer_new_timer(&timer_config, &gptimer_us);
gptimer_enable(gptimer_us);
gptimer_start(gptimer_us);
return gptimer_us;
}
int64_t tick_us(gptimer_handle_t gptimer_us, int64_t offset)
{
uint64_t count;
gptimer_get_raw_count(gptimer_us, &count);
return count + offset;
}
void app_main(void)
{
uart_driver_install(UART_NUM_2, 1024, 256, 0, NULL, 0);
uart_config_t uart_config = {
.baud_rate = 9600,
.data_bits = UART_DATA_8_BITS,
.parity = UART_PARITY_DISABLE,
.stop_bits = UART_STOP_BITS_1,
.flow_ctrl = UART_HW_FLOWCTRL_DISABLE,
.source_clk = UART_SCLK_DEFAULT};
uart_param_config(UART_NUM_2, &uart_config);
uart_set_pin(UART_NUM_2, 1, 2, -1, -1);
int n = 252;
char SendData[n];
for (int i = 0; i < n; i++)
{
SendData[i] = 'A';
}
SendData[n - 1] = 0;
gptimer_handle_t th = tick_us_start();
int64_t t = tick_us(th, 0);
uart_flush(UART_NUM_2);
uart_write_bytes(UART_NUM_2, SendData, sizeof(SendData));
printf("t= %f\n", (tick_us(th, 0) - t) / 1000.0);
}
Page 335 full program from fragment
#include <stdio.h>
#include "freertos/FreeRTOS.h"
#include "driver/uart.h"
#include "driver/gptimer.h"
gptimer_handle_t tick_us_start(void)
{
gptimer_config_t timer_config = {
.clk_src = GPTIMER_CLK_SRC_DEFAULT,
.direction = GPTIMER_COUNT_UP,
.resolution_hz = 1000000,
};
gptimer_handle_t gptimer_us = NULL;
gptimer_new_timer(&timer_config, &gptimer_us);
gptimer_enable(gptimer_us);
gptimer_start(gptimer_us);
return gptimer_us;
}
int64_t tick_us(gptimer_handle_t gptimer_us, int64_t offset)
{
uint64_t count;
gptimer_get_raw_count(gptimer_us, &count);
return count + offset;
}
void app_main(void)
{
uart_driver_install(UART_NUM_2, 1024, 256, 0, NULL, 0);
uart_config_t uart_config = {
.baud_rate = 9600,
.data_bits = UART_DATA_8_BITS,
.parity = UART_PARITY_DISABLE,
.stop_bits = UART_STOP_BITS_1,
.flow_ctrl = UART_HW_FLOWCTRL_DISABLE,
.source_clk = UART_SCLK_DEFAULT};
uart_param_config(UART_NUM_2, &uart_config);
uart_set_pin(UART_NUM_2, 1, 2, -1, -1);
int n = 1000;
char SendData[n];
for (int i = 0; i < n; i++)
{
SendData[i] = 'A';
}
SendData[n - 1] = 0;
gptimer_handle_t th = tick_us_start();
int64_t t = tick_us(th, 0);
uart_flush(UART_NUM_2);
uart_write_bytes(UART_NUM_2, SendData, sizeof(SendData));
printf("t= %f\n", (tick_us(th, 0) - t) / 1000.0);
char RecData[2000];
int nr = uart_read_bytes(UART_NUM_2, RecData,
sizeof(RecData), 300 / portTICK_PERIOD_MS);
RecData[nr + 1] = 0;
printf("%s\n", &RecData[1]);
printf("%d %d\n", nr, sizeof(SendData));
}
Page 335-336
#include "freertos/FreeRTOS.h"
#include <string.h>
#include <unistd.h>
#include "driver/uart.h"
#include "driver/gptimer.h"
gptimer_handle_t tick_us_start(void)
{
gptimer_config_t timer_config = {
.clk_src = GPTIMER_CLK_SRC_DEFAULT,
.direction = GPTIMER_COUNT_UP,
.resolution_hz = 1000000,
};
gptimer_handle_t gptimer_us = NULL;
gptimer_new_timer(&timer_config, &gptimer_us);
gptimer_enable(gptimer_us);
gptimer_start(gptimer_us);
return gptimer_us;
}
int64_t tick_us(gptimer_handle_t gptimer_us, int64_t offset)
{
uint64_t count;
gptimer_get_raw_count(gptimer_us, &count);
return count + offset;
}
void delay_us(int t)
{
usleep(t);
}
void app_main(void)
{
uart_driver_install(UART_NUM_2, 512, 1024 * 2, 0, NULL, 0);
uart_config_t uart_config = {
.baud_rate = 9600,
.data_bits = UART_DATA_8_BITS,
.parity = UART_PARITY_DISABLE,
.stop_bits = UART_STOP_BITS_1,
.flow_ctrl = UART_HW_FLOWCTRL_DISABLE,
.source_clk = UART_SCLK_DEFAULT};
uart_param_config(UART_NUM_2, &uart_config);
uart_set_pin(UART_NUM_2, 1, 2, -1, -1);
int n = 1000;
char SendData[n];
for (int i = 0; i < n; i++)
{
SendData[i] = 'A' + i / 40;
}
SendData[n - 1] = 0;
gptimer_handle_t th = tick_us_start();
int64_t t = tick_us(th, 0);
uart_flush(UART_NUM_2);
uart_write_bytes(UART_NUM_2, SendData, sizeof(SendData));
printf("t= %f\n", (tick_us(th, 0) - t) / 1000.0);
delay_us(2000 * 1000);
char RecData[2000];
int nr = uart_read_bytes(UART_NUM_2, RecData, sizeof(RecData),
300 / portTICK_PERIOD_MS);
RecData[nr + 1] = 0;
printf("%s\n", &RecData[1]);
printf("%d %d\n", nr, sizeof(SendData));
uart_driver_delete(UART_NUM_2);
}
Page 341
#include <stdio.h>
#include "freertos/FreeRTOS.h"
#include <unistd.h>
#include "driver/uart.h"
#include "driver/gptimer.h"
gptimer_handle_t tick_us_start(void)
{
gptimer_config_t timer_config = {
.clk_src = GPTIMER_CLK_SRC_DEFAULT,
.direction = GPTIMER_COUNT_UP,
.resolution_hz = 1000000,
};
gptimer_handle_t gptimer_us = NULL;
gptimer_new_timer(&timer_config, &gptimer_us);
gptimer_enable(gptimer_us);
gptimer_start(gptimer_us);
return gptimer_us;
}
int64_t tick_us(gptimer_handle_t gptimer_us, int64_t offset)
{
uint64_t count;
gptimer_get_raw_count(gptimer_us, &count);
return count + offset;
}
void delay_us(int t)
{
usleep(t);
}
void app_main(void)
{
uart_driver_install(UART_NUM_2, 256, 2000, 0, NULL, 0);
uart_config_t uart_config = {
.baud_rate = 9600,
.data_bits = UART_DATA_8_BITS,
.parity = UART_PARITY_DISABLE,
.stop_bits = UART_STOP_BITS_1,
.flow_ctrl = UART_HW_FLOWCTRL_CTS_RTS,
.rx_flow_ctrl_thresh = 50,
.source_clk = UART_SCLK_DEFAULT};
uart_param_config(UART_NUM_2, &uart_config);
uart_set_pin(UART_NUM_2, 1, 2, 4, 5);
int n = 1000;
char SendData[n];
for (int i = 0; i < n; i++)
{
SendData[i] = 'A' + i / 40;
}
SendData[n - 1] = 0;
gptimer_handle_t th = tick_us_start();
int64_t t = tick_us(th, 0);
uart_flush(UART_NUM_2);
uart_write_bytes(UART_NUM_2, SendData, sizeof(SendData));
printf("t= %f\n", (tick_us(th, 0) - t) / 1000.0);
fflush(stdout);
delay_us(1000 * 1000);
char RecData[2000];
int nr = 0;
int nt = 0;
do
{
nt = uart_read_bytes(UART_NUM_2, &RecData[nr],
sizeof(RecData) - nr, 50 / portTICK_PERIOD_MS);
nr = nr + nt;
printf("%d %d\n", nt, nr);
} while (nt != 0);
RecData[nr + 1] = 0;
printf("%s\n", &RecData[1]);
printf("%d %d\n", nr, sizeof(SendData));
uart_driver_delete(UART_NUM_2);
}
Page 347
#include <stdio.h>
#include "freertos/FreeRTOS.h"
#include <unistd.h>
#include "driver/uart.h"
void delay_us(int t)
{
usleep(t);
}
char uart_read(uart_port_t uart_num)
{
size_t num;
do
{
uart_get_buffered_data_len(uart_num, &num);
} while (num == 0);
char byte;
uart_read_bytes(UART_NUM_2, &byte, 1, 0);
return byte;
}
int uart_read_chars(uart_port_t uart_num, char *buf, int length, int timeout, int timeout_char)
{
int nr = uart_read_bytes(uart_num, buf, 1, timeout / portTICK_PERIOD_MS);
if (nr == 0)
return 0;
nr = uart_read_bytes(uart_num, &buf[1], length - 1, timeout_char / portTICK_PERIOD_MS);
return nr;
}
int presence(uart_port_t uart_num)
{
uart_set_baudrate(uart_num, 9600);
char byte = 0xF0;
uart_write_bytes(uart_num, &byte, 1);
delay_us(1000);
uart_read_bytes(uart_num, &byte, 1, 30 / portTICK_PERIOD_MS);
uart_set_baudrate(uart_num, 115200);
if (byte == 0xF0)
return -1;
return 0;
}
void writeByte(uart_port_t uart_num, int byte)
{
char buf[8];
for (int i = 0; i < 8; i++)
{
if ((byte & 1) == 1)
{
buf[i] = 0xFF;
}
else
{
buf[i] = 0x00;
}
byte = byte >> 1;
};
uart_write_bytes(uart_num, buf, 8);
delay_us(1000);
uart_read_bytes(uart_num, buf, 8, 30 / portTICK_PERIOD_MS);
}
char readByte(uart_port_t uart_num)
{
char buf[8];
for (int i = 0; i < 8; i++)
{
buf[i] = 0xFF;
}
uart_write_bytes(uart_num, buf, 8);
delay_us(1000);
uart_read_bytes(uart_num, buf, 8, 30 / portTICK_PERIOD_MS);
char result = 0;
for (int i = 0; i < 8; i++)
{
result = result >> 1;
if (buf[i] == 0xFF)
result = result | 0x80;
}
return result;
}
void app_main(void)
{
uart_driver_install(UART_NUM_2, 256, 2000, 0, NULL, 0);
uart_config_t uart_config = {
.baud_rate = 9600,
.data_bits = UART_DATA_8_BITS,
.parity = UART_PARITY_DISABLE,
.stop_bits = UART_STOP_BITS_1,
.flow_ctrl = UART_HW_FLOWCTRL_DISABLE,
.rx_flow_ctrl_thresh = 50,
.source_clk = UART_SCLK_DEFAULT};
uart_param_config(UART_NUM_2, &uart_config);
uart_set_pin(UART_NUM_2, 1, 2, -1, -1);
uart_flush(UART_NUM_2);
printf("%d\n", presence(UART_NUM_2));
presence(UART_NUM_2);
writeByte(UART_NUM_2, 0xCC);
writeByte(UART_NUM_2, 0x44);
delay_us(1000 * 1000);
presence(UART_NUM_2);
writeByte(UART_NUM_2, 0xCC);
writeByte(UART_NUM_2, 0xBE);
char data[9];
for (int i = 0; i < 9; i++)
{
data[i] = readByte(UART_NUM_2);
}
int t1 = data[0];
int t2 = data[1];
int temp1 = (t2 << 8 | t1);
if (t2 & 0x80)
temp1 = temp1 | 0xFFFF0000;
float temp = temp1 / 16.0;
printf("temp =%f\n", temp);
uart_driver_delete(UART_NUM_2);
}
Page 354 complete program from fragments
#include <stdio.h>
#include "freertos/FreeRTOS.h"
#include "nvs_flash.h"
#include "esp_wifi.h"
int retry_num = 0;
static void wifi_event_handler(void *event_handler_arg, esp_event_base_t event_base, int32_t event_id, void *event_data)
{
switch (event_id)
{
case WIFI_EVENT_STA_START:
printf("WIFI CONNECTING....\n");
break;
case WIFI_EVENT_STA_CONNECTED:
printf("WiFi CONNECTED\n");
break;
case WIFI_EVENT_STA_DISCONNECTED:
printf("WiFi lost connection\n");
if (retry_num < 5)
{
esp_wifi_connect();
retry_num++;
printf("Retrying to Connect...\n");
}
break;
case IP_EVENT_STA_GOT_IP:
printf("Wifi got IP...\n\n");
break;
}
}
void app_main(void)
{
nvs_flash_init();
esp_netif_init();
esp_event_loop_create_default();
esp_event_handler_register(WIFI_EVENT, ESP_EVENT_ANY_ID,
wifi_event_handler, NULL);
esp_event_handler_register(IP_EVENT, IP_EVENT_STA_GOT_IP,
wifi_event_handler, NULL);
wifi_init_config_t wificonfig = WIFI_INIT_CONFIG_DEFAULT();
esp_wifi_init(&wificonfig);
esp_netif_t *netif = esp_netif_create_default_wifi_sta();
wifi_config_t staconf = {
.sta = {
.ssid = "ssid",
.password = "password",
.threshold.authmode = WIFI_AUTH_WPA_PSK,
}};
esp_wifi_set_mode(WIFI_MODE_STA);
esp_wifi_set_config(WIFI_IF_STA, &staconf);
esp_wifi_set_country_code("CO", false);
esp_wifi_start();
esp_wifi_connect();
}
Page 355 wificonnect.h
int retry_num = 0;
int wifiStatus = 1000;
static void wifi_event_handler(void *event_handler_arg, esp_event_base_t event_base, int32_t event_id, void *event_data)
{
switch (event_id)
{
case WIFI_EVENT_STA_START:
wifiStatus = 1001;
break;
case WIFI_EVENT_STA_CONNECTED:
wifiStatus = 1002;
break;
case WIFI_EVENT_STA_DISCONNECTED:
if (retry_num < 5)
{
esp_wifi_connect();
retry_num++;
wifiStatus = 1001;
}
break;
case IP_EVENT_STA_GOT_IP:
wifiStatus = 1010;
break;
}
}
void wifiConnect(char *country, char *ssid, char *password)
{
nvs_flash_init();
esp_netif_init();
esp_event_loop_create_default();
esp_event_handler_register(WIFI_EVENT, ESP_EVENT_ANY_ID,
wifi_event_handler, NULL);
esp_event_handler_register(IP_EVENT, IP_EVENT_STA_GOT_IP,
wifi_event_handler, NULL);
wifi_init_config_t wificonfig = WIFI_INIT_CONFIG_DEFAULT();
esp_wifi_init(&wificonfig);
esp_netif_create_default_wifi_sta();
wifi_config_t staconf = {
.sta = {
.threshold.authmode = WIFI_AUTH_WPA_PSK}};
strcpy((char *)staconf.sta.ssid, ssid);
strcpy((char *)staconf.sta.password, password);
esp_wifi_set_mode(WIFI_MODE_STA);
esp_wifi_set_config(WIFI_IF_STA, &staconf);
esp_wifi_set_country_code(country, false);
esp_wifi_start();
esp_wifi_connect();
}
Page 356 main.c uses wificonnect.h
#include <stdio.h>
#include "freertos/FreeRTOS.h"
#include "esp_wifi.h"
#include "string.h"
#include "nvs_flash.h"
#include "wificonnect.h"
void app_main(void)
{
wifiConnect("co", "ssid", "password");
while (wifiStatus != 1010) {
vTaskDelay(10 / portTICK_PERIOD_MS);
};
//use WiFi connection
}
Page 361 uses wificonnect.h
#include <stdio.h>
#include "freertos/FreeRTOS.h"
#include "esp_wifi.h"
#include "nvs_flash.h"
#include "esp_event.h"
#include "esp_netif.h"
#include "string.h"
#include "socket.h"
#include "wificonnect.h"
void app_main(void)
{
wifiConnect("CO", "ssid", "password");
while (wifiStatus != 1010)
{
vTaskDelay(10 / portTICK_PERIOD_MS);
};
int sockfd = socket(AF_INET, SOCK_STREAM, 0);
struct sockaddr_in addr;
addr.sin_family = AF_INET;
addr.sin_port = htons(80);
addr.sin_addr.s_addr = 0x0Ed7b85d;
if (connect(sockfd, (struct sockaddr *)&addr, sizeof(addr)) < 0)
return;
char header[] = "GET /index.html HTTP/1.1\r\nHost:example.com\r\n\r\n";
int n = write(sockfd, header, strlen(header));
char buffer[2048];
n = read(sockfd, buffer, 2048);
buffer[n] = 0;
printf("%s\n", buffer);
}
Page 364 uses wificonnect.h
#include <stdio.h>
#include "freertos/FreeRTOS.h"
#include "esp_wifi.h"
#include "nvs_flash.h"
#include "esp_event.h"
#include "esp_netif.h"
#include "string.h"
#include "esp_http_client.h"
#include "wificonnect.h"
#include "esp_crt_bundle.h"
esp_err_t http_event_handler(esp_http_client_event_t *evt)
{
static int pos = 0;
switch (evt->event_id)
{
case HTTP_EVENT_ERROR:
printf("HTTP_EVENT_ERROR\n");
break;
case HTTP_EVENT_ON_CONNECTED:
printf("HTTP_EVENT_ON_CONNECTED\n");
break;
case HTTP_EVENT_HEADER_SENT:
printf("HTTP_EVENT_HEADER_SENT\n");
break;
case HTTP_EVENT_ON_HEADER:
printf("HTTP_EVENT_ON_HEADER\n");
printf("header = %s, %s\n", evt->header_key, evt->header_value);
break;
case HTTP_EVENT_ON_DATA:
printf("HTTP_EVENT_ON_DATA, len=%d\n", evt->data_len);
if (!esp_http_client_is_chunked_response(evt->client))
{
printf("%.*s", evt->data_len, (char *)evt->data);
char *buf = (char *)(evt->user_data);
memcpy(buf + pos, evt->data, evt->data_len);
pos += evt->data_len;
buf[pos] = 0;
}
break;
case HTTP_EVENT_ON_FINISH:
printf("HTTP_EVENT_ON_FINISH\n");
pos = 0;
break;
case HTTP_EVENT_DISCONNECTED:
printf("HTTP_EVENT_DISCONNECTED\n");
break;
default:
}
return ESP_OK;
}
char httpdata[2000];
void app_main(void)
{
wifiConnect("co", "ssid", "password");
while (wifiStatus != 1010)
{
vTaskDelay(10 / portTICK_PERIOD_MS);
};
esp_http_client_config_t httpconfig = {
.method = HTTP_METHOD_GET,
.event_handler = http_event_handler,
.buffer_size = DEFAULT_HTTP_BUF_SIZE,
.buffer_size_tx = DEFAULT_HTTP_BUF_SIZE,
.user_data = httpdata,
.transport_type = HTTP_TRANSPORT_OVER_SSL,
.crt_bundle_attach = esp_crt_bundle_attach,
};
esp_http_client_handle_t httphandle = esp_http_client_init(&httpconfig);
esp_http_client_perform(httphandle);
printf("len data= %d\n", strlen(httpdata));
printf("html \n %s\n ", httpdata);
}
Page 367 full program uses wificonnect.h
#include <stdio.h>
#include "freertos/FreeRTOS.h"
#include "esp_wifi.h"
#include "nvs_flash.h"
#include "esp_event.h"
#include "esp_netif.h"
#include "string.h"
#include "esp_http_client.h"
#include "wificonnect.h"
#include "esp_crt_bundle.h"
esp_err_t http_event_handler(esp_http_client_event_t *evt)
{
static int pos = 0;
switch (evt->event_id)
{
case HTTP_EVENT_ERROR:
printf("HTTP_EVENT_ERROR\n");
break;
case HTTP_EVENT_ON_CONNECTED:
printf("HTTP_EVENT_ON_CONNECTED\n");
break;
case HTTP_EVENT_HEADER_SENT:
printf("HTTP_EVENT_HEADER_SENT\n");
break;
case HTTP_EVENT_ON_HEADER:
printf("HTTP_EVENT_ON_HEADER\n");
printf("header = %s, %s\n", evt->header_key, evt->header_value);
break;
case HTTP_EVENT_ON_DATA:
printf("HTTP_EVENT_ON_DATA, len=%d\n", evt->data_len);
if (!esp_http_client_is_chunked_response(evt->client))
{
printf("%.*s", evt->data_len, (char *)evt->data);
char *buf = (char *)(evt->user_data);
memcpy(buf + pos, evt->data, evt->data_len);
pos += evt->data_len;
buf[pos] = 0;
}
break;
case HTTP_EVENT_ON_FINISH:
printf("HTTP_EVENT_ON_FINISH\n");
pos = 0;
break;
case HTTP_EVENT_DISCONNECTED:
printf("HTTP_EVENT_DISCONNECTED\n");
break;
default:
}
return ESP_OK;
}
char httpdata[2000];
void app_main(void)
{
wifiConnect("CO", "ssid", "password");
while (wifiStatus != 1010) {
vTaskDelay(10 / portTICK_PERIOD_MS);
};
esp_http_client_config_t httpconfig = {
.host = "192.168.253.75",
.path = "/test",
.port = 8080,
.method = HTTP_METHOD_PUT,
.event_handler = http_event_handler,
.buffer_size = DEFAULT_HTTP_BUF_SIZE,
.buffer_size_tx = DEFAULT_HTTP_BUF_SIZE,
.user_data = httpdata,
.transport_type = HTTP_TRANSPORT_OVER_TCP
};
esp_http_client_handle_t httphandle =
esp_http_client_init(&httpconfig);
esp_http_client_set_post_field(httphandle, "20.5", 3);
esp_http_client_perform(httphandle);
printf("html \n %s\n ", httpdata);
}
Page 368 Python
from http.server import HTTPServer, BaseHTTPRequestHandler
from io import BytesIO
class SimpleHTTPRequestHandler(BaseHTTPRequestHandler):
def log_message(self,*args, **kwargs):
pass
def do_PUT(self):
content_length = int(self.headers['Content-Length'])
body = self.rfile.read(content_length)
bodyString= body.decode(encoding="utf-8")
temp=float(bodyString)
print(temp)
self.send_response(200)
self.end_headers()
httpd = HTTPServer(('', 8080), SimpleHTTPRequestHandler)
httpd.serve_forever()
Page 368 complete program http server
#include <stdio.h>
#include "freertos/FreeRTOS.h"
#include "esp_wifi.h"
#include "nvs_flash.h"
#include "esp_event.h"
#include "esp_netif.h"
#include "string.h"
#include "wificonnect.h"
#include "esp_http_server.h"
esp_err_t get_handlertemp(httpd_req_t *req)
{
const char resp[] = "Temperature is 20.3";
httpd_resp_send(req, resp, HTTPD_RESP_USE_STRLEN);
return ESP_OK;
}
esp_err_t get_handlerhum(httpd_req_t *req)
{
/* Send a simple response */
const char resp[] = "Humidity is 80%";
httpd_resp_send(req, resp, HTTPD_RESP_USE_STRLEN);
return ESP_OK;
}
void app_main(void)
{
wifiConnect("co", "ssid", "password");
while (wifiStatus != 1010)
{
vTaskDelay(10 / portTICK_PERIOD_MS);
};
httpd_config_t config = HTTPD_DEFAULT_CONFIG();
httpd_handle_t server = NULL;
httpd_uri_t uri_get = {
.uri = "/temp",
.method = HTTP_GET,
.handler = get_handlertemp,
.user_ctx = NULL};
if (httpd_start(&server, &config) == ESP_OK)
{
httpd_register_uri_handler(server, &uri_get);
uri_get.uri = "/hum";
uri_get.handler = get_handlerhum;
httpd_register_uri_handler(server, &uri_get);
}
}
Page 371 Python
with open("iopress.key", 'rb') as f:
lines = f.readlines()
lines=b'"'.join(lines)
lines=lines.decode("ascii")
lines=lines.replace("\n",'\\n"\n')
print("static const unsigned char key[]="+'"', lines+";")
with open("iopress.crt", 'rb') as f:
lines = f.readlines()
lines=b'"'.join(lines)
lines=lines.decode("ascii")
lines=lines.replace("\n",'\\n"\n')
print("static const unsigned char cert[]="+'"', lines+";")
Page 372 HTTPS Server - the certificates listed will eventually timeout and they need to be replaced
#include <stdio.h>
#include "freertos/FreeRTOS.h"
#include "esp_wifi.h"
#include "nvs_flash.h"
#include "esp_event.h"
#include "esp_netif.h"
#include "string.h"
#include "wificonnect.h"
#include "esp_https_server.h"
esp_err_t get_handlertemp(httpd_req_t* req)
{
/* Send a simple response */
const char resp[] = "Temperature is 20.3";
httpd_resp_send(req, resp, HTTPD_RESP_USE_STRLEN);
return ESP_OK;
}
esp_err_t get_handlerhum(httpd_req_t* req)
{
/* Send a simple response */
const char resp[] = "Humidity is 80%";
httpd_resp_send(req, resp, HTTPD_RESP_USE_STRLEN);
return ESP_OK;
}
void app_main(void)
{
wifiConnect("co", "ssid", "password");
while (wifiStatus != 1010) {
vTaskDelay(10 / portTICK_PERIOD_MS);
};
static const unsigned char cert[] = " -----BEGIN CERTIFICATE-----\n"
"MIIDazCCAlOgAwIBAgIUA+lvUf9wMrNvaz9DuKnfx4TCoeQwDQYJKoZIhvcNAQEL\n"
"BQAwRTELMAkGA1UEBhMCR0IxEzARBgNVBAgMClNvbWUtU3RhdGUxITAfBgNVBAoM\n"
"GEludGVybmV0IFdpZGdpdHMgUHR5IEx0ZDAeFw0yNDA4MTYxMzQwMTNaFw0yNTA4\n"
"MTYxMzQwMTNaMEUxCzAJBgNVBAYTAkdCMRMwEQYDVQQIDApTb21lLVN0YXRlMSEw\n"
"HwYDVQQKDBhJbnRlcm5ldCBXaWRnaXRzIFB0eSBMdGQwggEiMA0GCSqGSIb3DQEB\n"
"AQUAA4IBDwAwggEKAoIBAQC5zxoZHid/tAtRY+V/Y1rRue4yMiHVLBTmh0kqGM/h\n"
"NvOuxJUnXKP2qn9cbM1OhZvF7NIIcqNTfHaNwDG+tF8p7YlQGcSBdk5+v3HTIAFI\n"
"gg3nwHEWdhNhfNnyHrjJG4YDkLGR9KZwMFfBYpsQJHwegUEpYG+5HnaMncjsJu2Q\n"
"bSO7fQ9dSBC7tIidfv6DhWdz/dHGjqpWYRwHhPACgwS1kKjWiOSrUMWUm3T3px7p\n"
"UfND7Ypz4/1ObTNZJs8zV8bnWp68YxS0rAeD0QIX3yTgvAGV56Dqdl2V4V5D/dpR\n"
"No99W3oUu99YeAK/t5pL+Xu5aXDdeg2e1OhPW7o9fZnlAgMBAAGjUzBRMB0GA1Ud\n"
"DgQWBBQQ4grGsqpNnsjZhWuWOg/Cey7AtTAfBgNVHSMEGDAWgBQQ4grGsqpNnsjZ\n"
"hWuWOg/Cey7AtTAPBgNVHRMBAf8EBTADAQH/MA0GCSqGSIb3DQEBCwUAA4IBAQAj\n"
"lw6xqyLBB86XIpW1YAINVHEw9x5ewMSbYTN7pDj01tRlaLfr8S4Qvo4FBA2Mq5fn\n"
"fstJzucb18yX15ZNo6xD1fAPYRf6BK6UwSeo/U4Hjewkk7gOyKEW8IjAMtWB5Svr\n"
"bZn4wxcSZEX/EHtGWe0kCZ4bDlWn9GuSjtAIZcrKo+jr0Cos2O4t19MWgxrbOwMx\n"
"AJYepL5+YcMd0NPuERBfTHE7mIG1heH8DAXAHtnFw26835aEyseZIR6EYPVxESYI\n"
"xmszSRgHFWDEqPpvaFpdOT1fT4KsloSP+wkE8DcmvjYRbPeabc4z8vTHRWgyLsHJ\n"
"mjqAoUl1y8um2Iw5ko0N\n"
"-----END CERTIFICATE-----\n"
;
static const unsigned char key[] = " -----BEGIN PRIVATE KEY-----\n"
"MIIEvQIBADANBgkqhkiG9w0BAQEFAASCBKcwggSjAgEAAoIBAQC5zxoZHid/tAtR\n"
"Y+V/Y1rRue4yMiHVLBTmh0kqGM/hNvOuxJUnXKP2qn9cbM1OhZvF7NIIcqNTfHaN\n"
"wDG+tF8p7YlQGcSBdk5+v3HTIAFIgg3nwHEWdhNhfNnyHrjJG4YDkLGR9KZwMFfB\n"
"YpsQJHwegUEpYG+5HnaMncjsJu2QbSO7fQ9dSBC7tIidfv6DhWdz/dHGjqpWYRwH\n"
"hPACgwS1kKjWiOSrUMWUm3T3px7pUfND7Ypz4/1ObTNZJs8zV8bnWp68YxS0rAeD\n"
"0QIX3yTgvAGV56Dqdl2V4V5D/dpRNo99W3oUu99YeAK/t5pL+Xu5aXDdeg2e1OhP\n"
"W7o9fZnlAgMBAAECggEABezu3iIyDEaHnd7bsMZQXSPazsr+fTfcqsVhte/4oSwJ\n"
"dWdbglfX+sPRL/dgTMLCBvvYbuCJCN6NQVQBwh0qc8HZgS5xL9fABRbB4IPCxrcv\n"
"Dlb6xEabs54xrSEBr5grG+3/W7I7pJRGGCq22zruomJo25LxvSuViEJ35+AN728d\n"
"rQW4qce1w0837O15u5EnWVrtEtFbvZHytuywvrGKDkB5mmoCE+31ASPnVH3iQFjw\n"
"s0HPmMufHfKRKnlucfCl5jJToHxIHf4JBd1LlvjpJfi0EtjLNbxg9vhCr4roKdqq\n"
"BvM+btu31X7Q/R0Ne3p7V5J3FRNwA3Bce7P6TVsqAQKBgQDdi+5e3FR8QY/qJ9xk\n"
"4MnxycBhcpd2Wtk7oqCs314fk0ifxtQHUh3QKPmTnqJiwxGRdYje05JHHCo2bgMb\n"
"FN7AHw1NsYSfsxqYxpgkovc1HJxKNbcqfI/bC1q1lT3Vt9gGk7DCD73EauVIAwOe\n"
"ybfpqte0Ej95zzTAWqRzw3JmQQKBgQDWtGiC/82qJ7X7xwpjnP0++RauF+TC71Hy\n"
"alqgTbwy0SEaGcxSdGMaxHKK0p94BIs3ZrSsSXLLUSrYC2c0UYnzFLT2i/uJD4NY\n"
"NrD4Xwq1Wo6vWLvY2EU618nTFmzDGOaC1enA030puRGRWEH+35iud7AIyuuPyLhr\n"
"Ek0zNIkypQKBgG9UUwu+QoJSW+R59WmIAFMNZCxT7kLeck1icsWMVXsegx8vRfsL\n"
"y8l/3bLNw6JHjjt/SbFXtikfwSKq88qXGTyIHiJNs2yhDxt4qJm4futknjE4fvvN\n"
"rmiPcxzOi00rXlYnv2o1iNH8OY2PXjFcApxcapqllNo8QrDqm7tEmudBAoGAcNua\n"
"CCoQYH3JQhSJGH1//OcQDekPXYxQ5f0TsCnMYGXfYYnoBfuZ0Issrl4yZvL0fuWk\n"
"2N8u0ULUI4Yy9KRbwAPFb8d7K7uUzfzJn3TN+zAjynX5H+3mzhx5wVSLTS48lM9+\n"
"tNY2d4UJf/4FisTby/Gr/aM0mXrnvZh8LgtShuUCgYEAw+3K2PalVraWHGbg18pz\n"
"fL212YObHDdbHM+SaBoopuiJed9Yz5DRbhVSSfuJMNu9WMjk9aR3Tr7s41l5L/+M\n"
"YGJGNJCE7I4mIfvgXwezxgd5P39+2Ei/qwR9nwsX/y6Mp3EuLKuPJUUaZERjrkIl\n"
"EVzn7XZ781QWSSBer5/vcQM=\n"
"-----END PRIVATE KEY-----\n"
;
httpd_ssl_config_t config = HTTPD_SSL_CONFIG_DEFAULT();
printf("%s", key);
config.servercert = cert;
config.servercert_len = sizeof(cert);
config.prvtkey_pem = key;
config.prvtkey_len = sizeof(key);
httpd_handle_t server = NULL;
httpd_uri_t uri_get = {
.uri = "/temp",
.method = HTTP_GET,
.handler = get_handlertemp,
.user_ctx = NULL
};
if (httpd_ssl_start(&server, &config) == ESP_OK) {
httpd_register_uri_handler(server, &uri_get);
uri_get.uri = "/hum";
uri_get.handler = get_handlerhum;
httpd_register_uri_handler(server, &uri_get);
}
}
Page 379
#include <stdio.h>
#include "freertos/FreeRTOS.h"
#include "driver/gpio.h"
void app_main(void)
{
uint32_t *GPIObase = (uint32_t *)0x60004000; // EP32 S3
// uint32_t* GPIObase = (uint32_t*)0x3FF44000; //ESP32
gpio_reset_pin(2);
gpio_set_direction(2, GPIO_MODE_OUTPUT);
uint32_t *GPIOSet = GPIObase + 8 / 4;
uint32_t *GPIOClear = GPIObase + 0xC / 4;
uint32_t mask = 1 << 2;
while (true)
{
*GPIOSet = mask;
vTaskDelay(1000 / portTICK_PERIOD_MS);
*GPIOClear = mask;
vTaskDelay(1000 / portTICK_PERIOD_MS);
}
}
Page 380
#include <stdio.h>
#include "driver/gpio.h"
#include "freertos/FreeRTOS.h"
#include "soc/gpio_reg.h"
void app_main(void)
{
gpio_reset_pin(2);
gpio_set_direction(2, GPIO_MODE_OUTPUT);
uint32_t mask = 1 << 2;
while (true)
{
*(int32_t *)GPIO_OUT_W1TS_REG = mask;
vTaskDelay(1000 / portTICK_PERIOD_MS);
*(int32_t *)GPIO_OUT_W1TC_REG = mask;
vTaskDelay(1000 / portTICK_PERIOD_MS);
}
}
Page 382
#include <stdio.h>
#include "driver/gpio.h"
#include "freertos/FreeRTOS.h"
#include "soc/gpio_reg.h"
void gpio_value_mask(int32_t value, int32_t mask)
{
*(int32_t *)GPIO_OUT_REG = (*(int32_t *)GPIO_OUT_REG & ~mask) |
(value & mask);
}
void app_main(void)
{
gpio_reset_pin(2);
gpio_set_direction(2, GPIO_MODE_OUTPUT);
gpio_reset_pin(4);
gpio_set_direction(4, GPIO_MODE_OUTPUT);
uint32_t mask = (1 << 2) | (1 << 4);
uint32_t value1 = (1 << 2);
uint32_t value2 = (1 << 4);
while (true)
{
gpio_value_mask(value1, mask);
gpio_value_mask(value2, mask);
}
}
Page 385 Complete program
#include <stdio.h>
#include "freertos/FreeRTOS.h"
#include "esp_rom_sys.h"
#include <sys/time.h>
#include "driver/ledc.h"
#include "math.h"
#include <unistd.h>
void delay_us(int t)
{
usleep(t);
}
bool isrollover(int timer)
{
int32_t *base = (int32_t *)0x600190C0; // ESP32 S3
// int32_t *base=(int32_t*)0x3FF59180; //ESP32
bool value = *((int32_t *)base) & 1 << timer;
*((int32_t *)base + 3) = 1 << timer;
return value;
}
void PWMconfigLow(int gpio, int chan, int timer, int res, int freq, float duty)
{
ledc_timer_config_t ledc_timer = {
.speed_mode = LEDC_LOW_SPEED_MODE,
.clk_cfg = LEDC_APB_CLK};
ledc_timer.timer_num = timer;
ledc_timer.duty_resolution = res;
ledc_timer.freq_hz = freq;
ledc_channel_config_t ledc_channel = {
.speed_mode = LEDC_LOW_SPEED_MODE,
.hpoint = 0,
.intr_type = LEDC_INTR_DISABLE,
};
ledc_channel.channel = chan;
ledc_channel.timer_sel = timer;
ledc_channel.gpio_num = gpio;
ledc_channel.duty = ((float)(2 << (res - 1))) * duty;
ledc_timer_config(&ledc_timer);
ledc_channel_config(&ledc_channel);
}
uint8_t wave[256];
void app_main(void)
{
gpio_reset_pin(4);
gpio_set_direction(4, GPIO_MODE_OUTPUT);
gpio_set_level(4, 0);
for (int i = 0; i < 256; i++)
{
wave[i] = (uint8_t)((128.0 + sinf((float)i * 2.0 *
3.14159 / 255.0) *
128.0));
}
int f = 60000;
PWMconfigLow(2, 0, 0, 8, f, 0.25);
while (true)
{
for (int i = 0; i < 256; i++)
{
ledc_set_duty(LEDC_LOW_SPEED_MODE, 0, wave[i]);
ledc_update_duty(LEDC_LOW_SPEED_MODE, 0);
while (!isrollover(0))
{
};
}
}
}
Page 385 full program from fragment
#include "esp_wifi.h"
#include "string.h"
#include "nvs_flash.h"
#include "esp_netif_sntp.h"
#include "sys/time.h"
#include "wificonnect.h"
void app_main(void)
{
wifiConnect("co", "ssid", "password");
while (wifiStatus != 1010)
{
vTaskDelay(3 / portTICK_PERIOD_MS);
};
esp_sntp_config_t config = ESP_NETIF_SNTP_DEFAULT_CONFIG("pool.ntp.org");
esp_netif_sntp_init(&config);
if (esp_netif_sntp_sync_wait(pdMS_TO_TICKS(10000)) != ESP_OK)
{
printf("Failed to update system time within 10s timeout");
}
struct timeval t;
gettimeofday(&t, NULL);
setenv("TZ", "UTC-1", 1);
tzset();
struct tm *tm = localtime(&(t.tv_sec));
printf("hour = %d\n", tm->tm_hour);
printf("min = %d\n", tm->tm_min);
char date[100];
strftime(date, 100, "%a, %d %b %Y %T", tm);
printf("date = %s\n", date);
}
Page 390
#include <stdio.h>
#include "freertos/FreeRTOS.h"
#include "esp_sleep.h"
void app_main(void)
{
esp_sleep_enable_timer_wakeup(1000000);
for (int i = 0; i < 10; i++)
{
printf("starting sleep %d \n", i);
fflush(stdout);
esp_light_sleep_start();
printf("back from sleep %d \n", i);
fflush(stdout);
}
}
Page 390
#include <stdio.h>
#include "freertos/FreeRTOS.h"
#include "esp_sleep.h"
void app_main(void)
{
esp_sleep_enable_timer_wakeup(1000000);
for (int i = 0; i < 10; i++)
{
printf("starting sleep %d \n", i);
fflush(stdout);
esp_deep_sleep_start();
printf("back from sleep %d \n", i);
fflush(stdout);
}
}
Page 399
#include <stdio.h>
#include "freertos/FreeRTOS.h"
#include "esp_partition.h"
void app_main(void)
{
esp_partition_iterator_t partit = esp_partition_find(ESP_PARTITION_TYPE_DATA, ESP_PARTITION_SUBTYPE_ANY, NULL);
while (partit != NULL)
{
const esp_partition_t *part = esp_partition_get(partit);
printf("DATA label= %s Address= %lX size = %ld \n", part->label, part->address, part->size);
partit = esp_partition_next(partit);
};
esp_partition_iterator_release(partit);
printf("\n");
partit = esp_partition_find(ESP_PARTITION_TYPE_APP, ESP_PARTITION_SUBTYPE_ANY, NULL);
while (partit != NULL)
{
const esp_partition_t *part = esp_partition_get(partit);
printf("APP label= %s Address= %lX size = %ld \n", part->label, part->address, part->size);
partit = esp_partition_next(partit);
};
esp_partition_iterator_release(partit);
return;
}
Page 403
#include <stdio.h>
#include "freertos/FreeRTOS.h"
#include "nvs_flash.h"
void app_main(void)
{
nvs_flash_init();
nvs_handle_t my_handle;
nvs_open("localstorage", NVS_READWRITE, &my_handle);
char key[] = "mykey";
int32_t myvalue = 42;
int32_t myretrievedvalue;
nvs_set_i32(my_handle, key, myvalue);
nvs_commit(my_handle);
nvs_get_i32(my_handle, key, &myretrievedvalue);
printf("%ld\n", myretrievedvalue);
return;
}
Page 404 You have to create a data partition subtype fat named FAT for this to work
#include <stdio.h>
#include "freertos/FreeRTOS.h"
#include "esp_vfs_fat.h"
static wl_handle_t s_wl_handle = WL_INVALID_HANDLE;
void app_main(void)
{
const esp_vfs_fat_mount_config_t mount_config = {
.max_files = 4,
.format_if_mount_failed = true,
.allocation_unit_size = CONFIG_WL_SECTOR_SIZE,
};
esp_err_t err = esp_vfs_fat_spiflash_mount_rw_wl("/spiflash", "FAT", &mount_config, &s_wl_handle);
if (err != ESP_OK) {
printf("%X\n",err);
return;
}
FILE *f = fopen("/spiflash/hello.txt", "wb");
fprintf(f, "Hello world");
fclose(f);
char buf[25];
f = fopen("/spiflash/hello.txt", "rb");
fgets(buf,sizeof(buf),f);
fclose(f);
printf("%s\n",buf);
return;
}
Page 408
#include <string.h>
#include "esp_vfs_fat.h"
#include "sdmmc_cmd.h"
void app_main(void)
{
spi_bus_config_t bus_cfg = {
.mosi_io_num = 15,
.miso_io_num = 4,
.sclk_io_num = 18,
.quadwp_io_num = -1,
.quadhd_io_num = -1,
.max_transfer_sz = 4000,
};
sdmmc_host_t host_config = SDSPI_HOST_DEFAULT();
host_config.max_freq_khz = 400;
sdspi_device_config_t slot_config =
SDSPI_DEVICE_CONFIG_DEFAULT();
slot_config.gpio_cs = 5;
slot_config.host_id = host_config.slot;
esp_vfs_fat_sdmmc_mount_config_t mount_config = {
.format_if_mount_failed = false,
.max_files = 5,
.allocation_unit_size = 16 * 1024
};
spi_bus_initialize(host_config.slot, &bus_cfg,
SDSPI_DEFAULT_DMA);
sdmmc_card_t* card;
esp_err_t err = esp_vfs_fat_sdspi_mount("/sdcard",
&host_config, &slot_config, &mount_config, &card);
if (err != ESP_OK) {
printf("%d", err);
}
sdmmc_card_print_info(stdout, card);
FILE *f = fopen("/sdcard/hello.txt", "w");
fprintf(f, "Hello World");
fclose(f);
char buf[25];
f = fopen("/sdcard/hello.txt", "rb");
fgets(buf,sizeof(buf),f);
fclose(f);
printf("%s\n",buf);
return;
}
Page 416
#include <stdio.h>
#include "freertos/FreeRTOS.h"
#include "driver/gpio.h"
void task1(void *arg)
{
for (;;)
{
gpio_set_level(2, 1);
}
}
void task2(void *arg)
{
for (;;)
{
gpio_set_level(2, 0);
}
}
void app_main(void)
{
gpio_reset_pin(2);
gpio_set_direction(2, GPIO_MODE_OUTPUT);
TaskHandle_t th1;
xTaskCreatePinnedToCore(task1, "task1", 2048,
NULL, 0, &th1, 1);
TaskHandle_t th2;
xTaskCreatePinnedToCore(task2, "task2", 2048,
NULL, 0, &th2, 1);
}
Page 422
#include <stdio.h>
#include "freertos/FreeRTOS.h"
uint64_t flag1 = 0;
uint64_t flag2 = 0;
void task1(void *arg)
{
for (;;)
{
flag1 = 0xFFFFFFFFFFFFFFFF;
flag2 = 0xFFFFFFFFFFFFFFFF;
if (flag1 != flag2)
{
printf("task 1 %llX %llX\n", flag1, flag2);
fflush(stdout);
}
}
}
void task2(void *arg)
{
for (;;)
{
flag1 = 0x0;
flag2 = 0x0;
if (flag1 != flag2)
{
printf("task 2 %llX %llX\n", flag1, flag1);
fflush(stdout);
}
}
}
void app_main(void)
{
TaskHandle_t th1;
xTaskCreatePinnedToCore(task1, "task1", 4048, NULL, 0, &th1, 1);
TaskHandle_t th2;
xTaskCreatePinnedToCore(task2, "task2", 4048, NULL, 0, &th2, 0);
}
Path 424
#include <stdio.h>
#include "freertos/FreeRTOS.h"
int64_t count = 0;
void task1(void *arg)
{
for (int i = 0; i < 0xFFFFF; i++)
{
count = count + 1;
}
for (;;)
{
}
}
void task2(void *arg)
{
for (int i = 0; i < 0xFFFFF; i++)
{
count = count + 1;
}
for (;;)
{
}
}
void app_main(void)
{
TaskHandle_t th1;
xTaskCreatePinnedToCore(task1, "task1", 2048, NULL, 0, &th1, 1);
TaskHandle_t th2;
xTaskCreatePinnedToCore(task2, "task2", 2048, NULL, 0, &th2, 0);
vTaskDelay(4000 / portTICK_PERIOD_MS);
printf("%llX\n", count);
}
Path 426
#include <stdio.h>
#include "freertos/FreeRTOS.h"
int64_t count = 0;
static portMUX_TYPE my_spinlock = portMUX_INITIALIZER_UNLOCKED;
void task1(void *arg)
{
for (int i = 0; i < 0xFFFFF; i++)
{
taskENTER_CRITICAL(&my_spinlock);
count = count + 1;
taskEXIT_CRITICAL(&my_spinlock);
}
for (;;)
{
}
}
void task2(void *arg)
{
for (int i = 0; i < 0xFFFFF; i++)
{
taskENTER_CRITICAL(&my_spinlock);
count = count + 1;
taskEXIT_CRITICAL(&my_spinlock);
}
for (;;)
{
}
}
void app_main(void)
{
TaskHandle_t th1;
xTaskCreatePinnedToCore(task1, "task1", 2048, NULL, 0, &th1, 1);
TaskHandle_t th2;
xTaskCreatePinnedToCore(task2, "task2", 2048, NULL, 0, &th2, 0);
vTaskDelay(4000 / portTICK_PERIOD_MS);
printf("%llX\n", count);
}
Page 428
#include <stdio.h>
#include "freertos/FreeRTOS.h"
QueueHandle_t q;
int64_t count = 0;
void task1(void *arg)
{
for (;;)
{
xQueueSendToBack(q, &count, 2);
count++;
vTaskDelay(1);
}
}
void task2(void *arg)
{
int64_t data;
for (;;)
{
xQueueReceive(q, &data, 20);
printf("%llX %d\n", data, uxQueueSpacesAvailable(q));
}
}
void app_main(void)
{
q = xQueueCreate(100, sizeof(int64_t));
TaskHandle_t th1;
xTaskCreatePinnedToCore(task1, "task1", 2048, NULL, 0, &th1, 1);
TaskHandle_t th2;
xTaskCreatePinnedToCore(task2, "task2", 4048, NULL, 0, &th2, 0);
}